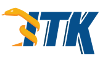 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkStatisticsAlgorithm_h
19 #define itkStatisticsAlgorithm_h
29 template <
typename TSize>
33 template <
typename TValue>
35 MedianOfThree(
const TValue a,
const TValue b,
const TValue c);
52 template <
typename TSample>
55 const typename TSample::ConstIterator & begin,
56 const typename TSample::ConstIterator & end,
57 typename TSample::MeasurementVectorType & min,
58 typename TSample::MeasurementVectorType & max);
61 template <
typename TSubsample>
66 typename TSubsample::MeasurementVectorType & min,
67 typename TSubsample::MeasurementVectorType & max,
68 typename TSubsample::MeasurementVectorType & mean);
91 template <
typename TSubsample>
94 unsigned int activeDimension,
97 const typename TSubsample::MeasurementType partitionValue);
116 template <
typename TSubsample>
117 typename TSubsample::MeasurementType
119 unsigned int activeDimension,
123 typename TSubsample::MeasurementType medianGuess);
139 template <
typename TSubsample>
140 typename TSubsample::MeasurementType
141 QuickSelect(TSubsample * sample,
unsigned int activeDimension,
int beginIndex,
int endIndex,
int kth);
151 template <
typename TSubsample>
152 typename TSubsample::MeasurementType
153 NthElement(TSubsample * sample,
unsigned int activeDimension,
int beginIndex,
int endIndex,
int nth);
155 template <
typename TSubsample>
157 InsertSort(TSubsample * sample,
unsigned int activeDimension,
int beginIndex,
int endIndex);
159 template <
typename TSubsample>
161 DownHeap(TSubsample * sample,
unsigned int activeDimension,
int beginIndex,
int endIndex,
int node);
163 template <
typename TSubsample>
165 HeapSort(TSubsample * sample,
unsigned int activeDimension,
int beginIndex,
int endIndex);
167 template <
typename TSubsample>
170 unsigned int activeDimension,
176 template <
typename TSubsample>
178 IntrospectiveSort(TSubsample * sample,
unsigned int activeDimension,
int beginIndex,
int endIndex,
int sizeThreshold);
184 #ifndef ITK_MANUAL_INSTANTIATION
185 # include "itkStatisticsAlgorithm.hxx"
188 #endif // #ifndef itkStatisticsAlgorithm_h
int Partition(TSubsample *sample, unsigned int activeDimension, int beginIndex, int endIndex, const typename TSubsample::MeasurementType partitionValue)
void IntrospectiveSort(TSubsample *sample, unsigned int activeDimension, int beginIndex, int endIndex, int sizeThreshold)
TSize FloorLog(TSize size)
void InsertSort(TSubsample *sample, unsigned int activeDimension, int beginIndex, int endIndex)
void IntrospectiveSortLoop(TSubsample *sample, unsigned int activeDimension, int beginIndex, int endIndex, int depthLimit, int sizeThreshold)
void FindSampleBound(const TSample *sample, const typename TSample::ConstIterator &begin, const typename TSample::ConstIterator &end, typename TSample::MeasurementVectorType &min, typename TSample::MeasurementVectorType &max)
void FindSampleBoundAndMean(const TSubsample *sample, int beginIndex, int endIndex, typename TSubsample::MeasurementVectorType &min, typename TSubsample::MeasurementVectorType &max, typename TSubsample::MeasurementVectorType &mean)
TSubsample::MeasurementType QuickSelect(TSubsample *sample, unsigned int activeDimension, int beginIndex, int endIndex, int kth, typename TSubsample::MeasurementType medianGuess)
TSubsample::MeasurementType NthElement(TSubsample *sample, unsigned int activeDimension, int beginIndex, int endIndex, int nth)
void HeapSort(TSubsample *sample, unsigned int activeDimension, int beginIndex, int endIndex)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
void DownHeap(TSubsample *sample, unsigned int activeDimension, int beginIndex, int endIndex, int node)
TValue MedianOfThree(const TValue a, const TValue b, const TValue c)