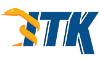 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkArithmeticOpsFunctors_h
19 #define itkArithmeticOpsFunctors_h
32 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
33 class ITK_TEMPLATE_EXPORT
Add2
42 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Add2);
47 return static_cast<TOutput>(A + B);
57 template <
typename TInput1,
typename TInput2,
typename TInput3,
typename TOutput>
58 class ITK_TEMPLATE_EXPORT
Add3
67 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Add3);
70 operator()(
const TInput1 & A,
const TInput2 & B,
const TInput3 & C)
const
72 return static_cast<TOutput>(A + B + C);
82 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
83 class ITK_TEMPLATE_EXPORT
Sub2
92 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Sub2);
97 return static_cast<TOutput>(A - B);
107 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
117 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Mult);
122 return static_cast<TOutput>(A * B);
132 template <
typename TInput1,
typename TInput2,
typename TOutput>
133 class ITK_TEMPLATE_EXPORT
Div
142 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Div);
149 return (TOutput)(A / B);
164 template <
typename TNumerator,
typename TDenominator = TNumerator,
typename TOutput = TNumerator>
171 m_Constant = TOutput{};
188 operator()(
const TNumerator & n,
const TDenominator & d)
const
194 return static_cast<TOutput>(n) / static_cast<TOutput>(d);
206 template <
typename TInput1,
typename TInput2,
typename TOutput>
216 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Modulus);
223 return static_cast<TOutput>(A % B);
232 #if !defined(ITK_FUTURE_LEGACY_REMOVE)
242 template <
typename TInput,
typename TOutput>
243 class ITK_TEMPLATE_EXPORT ModulusTransform
246 ModulusTransform() { m_Dividend = 5; }
247 ~ModulusTransform() =
default;
249 SetDividend(TOutput dividend)
251 m_Dividend = dividend;
256 operator==(
const ModulusTransform & other)
const
258 return m_Dividend == other.m_Dividend;
261 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(ModulusTransform);
264 operator()(
const TInput & x)
const
266 auto result = static_cast<TOutput>(x % m_Dividend);
286 template <
class TInput1,
class TInput2,
class TOutput>
301 const double temp = std::floor(static_cast<double>(A) / static_cast<double>(B));
302 if (std::is_integral_v<TOutput> && Math::isinf(temp))
313 return static_cast<TOutput>(temp);
328 template <
class TInput1,
class TInput2,
class TOutput>
355 template <
class TInput1,
class TOutput = TInput1>
370 return (TOutput)(-A);
TOutput operator()(const TInput1 &A, const TInput2 &B) const
TOutput operator()(const TInput1 &A) const
bool operator==(const UnaryMinus &) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const
static constexpr T NonpositiveMin()
bool operator==(const Div &) const
ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(DivFloor)
bool operator==(const Add2 &) const
bool operator==(const DivideOrZeroOut &) const
bool operator==(const Add3 &) const
TOutput operator()(const TNumerator &n, const TDenominator &d) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const
TOutput operator()(const TInput1 &A, const TInput2 &B, const TInput3 &C) const
bool NotAlmostEquals(T1 x1, T2 x2)
ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(UnaryMinus)
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
TOutput operator()(const TInput1 &A, const TInput2 &B) const
Cast arguments to double, performs division then takes the floor.
TOutput operator()(const TInput1 &A, const TInput2 &B) const
bool operator==(const Mult &) const
Define additional traits for native types such as int or float.
static constexpr T max(const T &)
bool operator==(const Modulus &) const
Promotes arguments to real type and performs division.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Apply the unary minus operator.
ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(DivReal)
static constexpr double e
TOutput operator()(const TInput1 &A, const TInput2 &B) const
bool operator==(const DivFloor &) const
bool operator==(const Sub2 &) const
bool operator==(const DivReal &) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const