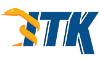 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
28 #ifndef itkConceptChecking_h
29 #define itkConceptChecking_h
36 #ifndef ITK_CONCEPT_NO_CHECKING
37 # if defined(_MSC_VER) && !defined(__ICL)
38 # define ITK_CONCEPT_IMPLEMENTATION_VTABLE
42 # define ITK_CONCEPT_IMPLEMENTATION_STANDARD
47 #if defined(ITK_CONCEPT_IMPLEMENTATION_STANDARD)
59 # define itkConceptConstraintsMacro() \
60 template <void (Constraints::*)()> \
63 using EnforcerInstantiation = Enforcer<&Constraints::constraints>; \
64 ITK_MACROEND_NOOP_STATEMENT
65 # define itkConceptMacro(name, concept) \
68 name = sizeof concept \
70 ITK_MACROEND_NOOP_STATEMENT
72 #elif defined(ITK_CONCEPT_IMPLEMENTATION_VTABLE)
79 # define itkConceptConstraintsMacro() \
80 virtual void Enforcer() { &Constraints::constraints; }
81 # define itkConceptMacro(name, concept) \
84 name = sizeof concept \
88 #elif defined(ITK_CONCEPT_IMPLEMENTATION_CALL)
91 # define itkConceptConstraintsMacro()
92 # define itkConceptMacro(name, concept) \
101 # define itkConceptConstraintsMacro()
102 # define itkConceptMacro(name, concept) \
126 template <
typename T>
132 template <
unsigned int>
144 template <
typename T>
153 template <
typename T>
164 template <
typename T>
182 template <
typename T>
215 template <
typename T1,
typename T2>
223 auto b = static_cast<T2>(
a);
234 template <
typename T>
261 template <
typename T1,
typename T2 = T1>
283 template <
typename T1,
typename T2 = T1>
305 template <
typename T1,
typename T2 = T1>
314 ITK_GCC_SUPPRESS_Wfloat_equal
330 template <
typename T1,
typename T2 = T1>
343 ITK_GCC_SUPPRESS_Wfloat_equal
359 template <
typename T1,
typename T2 = T1,
typename T3 = T1>
367 a = static_cast<T3>(
b +
c);
368 a = static_cast<T3>(
b -
c);
376 a = static_cast<T3>(d +
e);
377 a = static_cast<T3>(d -
e);
391 template <
typename T1,
typename T2 = T1>
420 template <
typename T1,
typename T2 = T1,
typename T3 = T1>
428 a = static_cast<T3>(
b *
c);
436 a = static_cast<T3>(d *
e);
447 template <
typename T1,
typename T2 = T1>
474 template <
typename T1,
typename T2 = T1,
typename T3 = T1>
482 a = static_cast<T3>(
b /
c);
490 a = static_cast<T3>(d /
e);
503 template <
typename T1,
typename T2 = T1>
532 template <
typename T1,
typename T2 = T1,
typename T3 = T1>
540 a = static_cast<T3>(
b &
c);
541 a = static_cast<T3>(
b |
c);
542 a = static_cast<T3>(
b ^
c);
543 a &= static_cast<T3>(
c);
544 a |= static_cast<T3>(
c);
545 a ^= static_cast<T3>(
c);
553 a = static_cast<T3>(d &
e);
554 a = static_cast<T3>(d |
e);
555 a = static_cast<T3>(d ^
e);
556 a &= static_cast<T3>(
e);
557 a |= static_cast<T3>(
e);
558 a ^= static_cast<T3>(
e);
570 template <
typename T1,
typename T2 = T1,
typename T3 = T1>
578 a = static_cast<T3>(
b[
c]);
586 a = static_cast<T3>(d[
e]);
598 template <
typename T>
616 template <
typename T>
637 template <
typename T>
655 template <
typename T>
678 template <
typename T1,
typename T2>
695 template <
unsigned int D1,
unsigned int D2>
715 template <
typename T>
752 template <
typename T>
771 template <
typename T>
788 template <
typename T>
807 template <
typename T1,
typename T2>
824 template <
unsigned int D1,
unsigned int D2>
848 template <
unsigned int D1,
unsigned int D2>
876 template <
typename T>
880 static constexpr
bool Integral = std::is_integral_v<T>;
900 template <
typename T>
924 template <
typename T>
947 template <
typename T>
951 static constexpr
bool Integral = std::is_integral_v<T>;
952 static constexpr
bool IsExact = std::numeric_limits<typename NumericTraits<T>::ValueType>::is_exact;
974 template <
typename T>
978 static constexpr
bool Integral = std::is_integral_v<T>;
979 static constexpr
bool IsExact = std::numeric_limits<typename NumericTraits<T>::ValueType>::is_exact;
itkConceptConstraintsMacro()
static constexpr bool Unsigned
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
static bool IsPositive(T val)
itkConceptConstraintsMacro()
Detail::UniqueType_unsigned_int< D2 > DT2
Detail::UniqueType_bool< false > FalseT
static constexpr bool IsExact
void const_constraints(const T1 &d, const T2 &e)
Detail::UniqueType_bool< false > FalseT
static constexpr bool Integral
itkConceptConstraintsMacro()
static constexpr T NonpositiveMin()
itkConceptConstraintsMacro()
Detail::UniqueType_bool< false > FalseT
itkConceptConstraintsMacro()
void const_constraints(const T &a)
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
void const_constraints(const T1 &d, const T2 &e)
Traits for a pixel that define the dimension and component type.
itkConceptConstraintsMacro()
Detail::UniqueType_bool< true > TrueT
void const_constraints(const T1 &d)
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
void const_constraints(const T1 &d)
itkConceptConstraintsMacro()
void const_constraints(const T &b)
static constexpr bool IsSigned
itkConceptConstraintsMacro()
Detail::UniqueType_bool< true > TrueT
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
void const_constraints(const T1 &d, const T2 &e)
void const_constraints(const T1 &d)
void const_constraints(const T1 &d, const T2 &e)
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
void f(Type3, int=0, int=0)
itkConceptConstraintsMacro()
Define additional traits for native types such as int or float.
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
Detail::UniqueType_bool< true > TrueT
static bool IsNonnegative(T val)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
itkConceptConstraintsMacro()
void const_constraints(const T1 &d, const T2 &e)
static bool IsNonpositive(T val)
static constexpr bool Integral
itkConceptConstraintsMacro()
Detail::UniqueType_bool< true > TrueT
static constexpr bool IsExact
static constexpr double e
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
void RequireBooleanExpression(const T &t)
static constexpr bool Integral
static bool IsNegative(T val)
static constexpr bool NonIntegral
itkConceptConstraintsMacro()
void IgnoreUnusedVariable(T)