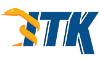 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef VNLSparseLUSolverTraits_h
19 #define VNLSparseLUSolverTraits_h
21 #include "vnl/vnl_vector.h"
22 #include "vnl/vnl_sparse_matrix.h"
23 #include "vnl/algo/vnl_sparse_lu.h"
41 template <
typename T =
double>
97 Solve(solver, iB, oX);
114 Solve(solver, iBx, iBy, iBz, oX, oY, oZ);
124 Solve(solver, iBx, iBy, oX, oY);
133 oX = solver.solve(iB);
147 oX = solver.solve(iBx);
148 oY = solver.solve(iBy);
149 oZ = solver.solve(iBz);
157 oX = solver.solve(iBx);
158 oY = solver.solve(iBy);
vnl_sparse_matrix< ValueType > MatrixType
vnl_vector< ValueType > VectorType
static void Solve(SolverType &solver, const VectorType &iBx, const VectorType &iBy, const VectorType &iBz, VectorType &oX, VectorType &oY, VectorType &oZ)
Solve the linear systems: , , factoring the internal matrix if needed.
static void FillMatrix(MatrixType &iA, const unsigned int &iR, const unsigned int &iC, const ValueType &iV)
iA[iR][iC] = iV
static bool Solve(const MatrixType &iA, const VectorType &iBx, const VectorType &iBy, VectorType &oX, VectorType &oY)
Solve the linear systems: , .
static bool IsDirectSolver()
static VectorType InitializeVector(const unsigned int &iN)
initialize a vector of size iN
static bool Solve(const MatrixType &iA, const VectorType &iBx, const VectorType &iBy, const VectorType &iBz, VectorType &oX, VectorType &oY, VectorType &oZ)
Solve the linear systems: , , .
static MatrixType InitializeSparseMatrix(const unsigned int &iN)
initialize a square sparse matrix of size iN x iN
static MatrixType InitializeSparseMatrix(const unsigned int &iRow, const unsigned int &iCol)
initialize a sparse matrix of size iRow x iCol
static void AddToMatrix(MatrixType &iA, const unsigned int &iR, const unsigned int &iC, const ValueType &iV)
iA[iR][iC] += iV
static void Solve(SolverType &solver, const VectorType &iBx, const VectorType &iBy, VectorType &oX, VectorType &oY)
Solve the linear systems: , factoring the internal matrix if needed.
static void Solve(SolverType &solver, const VectorType &iB, VectorType &oX)
Solve the linear system factoring the internal matrix if needed.
static bool Solve(const MatrixType &iA, const VectorType &iB, VectorType &oX)
Solve the linear system .
Generic interface for sparse LU solver.