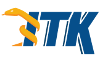 |
ITK
5.1.0
Insight Toolkit
|
Go to the documentation of this file.
47 ITK_DISALLOW_COPY_AND_ASSIGN(
Command);
111 m_MemberFunction = memberFunction;
118 m_ConstMemberFunction = memberFunction;
125 if (m_MemberFunction)
127 ((*m_This).*(m_MemberFunction))(caller, event);
136 if (m_ConstMemberFunction)
138 ((*m_This).*(m_ConstMemberFunction))(caller, event);
150 , m_MemberFunction(nullptr)
151 , m_ConstMemberFunction(nullptr)
166 template <
typename T>
191 m_MemberFunction = memberFunction;
198 if (m_MemberFunction)
200 ((*m_This).*(m_MemberFunction))(event);
209 if (m_MemberFunction)
211 ((*m_This).*(m_MemberFunction))(event);
222 , m_MemberFunction(nullptr)
237 template <
typename T>
261 m_MemberFunction = memberFunction;
268 if (m_MemberFunction)
270 ((*m_This).*(m_MemberFunction))();
278 if (m_MemberFunction)
280 ((*m_This).*(m_MemberFunction))();
290 , m_MemberFunction(nullptr)
305 template <
typename T>
329 m_MemberFunction = memberFunction;
336 if (m_MemberFunction)
338 ((*m_This).*(m_MemberFunction))();
346 if (m_MemberFunction)
348 ((*m_This).*(m_MemberFunction))();
358 , m_MemberFunction(nullptr)
398 SetClientData(
void * cd);
423 void * m_ClientData{
nullptr };
void(T::*)() TMemberFunctionPointer
void SetCallbackFunction(T *object, TConstMemberFunctionPointer memberFunction)
A Command subclass that calls a pointer to a member function.
void SetCallbackFunction(const T *object, TMemberFunctionPointer memberFunction)
TMemberFunctionPointer m_MemberFunction
void(T::*)(const Object *, const EventObject &) TConstMemberFunctionPointer
void Execute(Object *, const EventObject &) override
A Command subclass that calls a pointer to a member function.
void(*)(const Object *, const EventObject &, void *) ConstFunctionPointer
void Execute(const Object *caller, const EventObject &event) override
A Command subclass that calls a pointer to a member function.
void Execute(const Object *, const EventObject &) override
void(*)(void *) DeleteDataFunctionPointer
void Execute(Object *, const EventObject &event) override
Superclass for callback/observer methods.
void Execute(const Object *, const EventObject &event) override
void Execute(Object *caller, const EventObject &event) override
SimpleConstMemberCommand()
class ITK_FORWARD_EXPORT Command
void Execute(const Object *, const EventObject &) override
TMemberFunctionPointer m_MemberFunction
TConstMemberFunctionPointer m_ConstMemberFunction
void Execute(Object *, const EventObject &) override
void SetCallbackFunction(T *object, TMemberFunctionPointer memberFunction)
A Command subclass that calls a pointer to a member function.
void SetCallbackFunction(T *object, TMemberFunctionPointer memberFunction)
TMemberFunctionPointer m_MemberFunction
void(T::*)() const TMemberFunctionPointer
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
void SetCallbackFunction(T *object, TMemberFunctionPointer memberFunction)
void(*)(Object *, const EventObject &, void *) FunctionPointer
TMemberFunctionPointer m_MemberFunction
Base class for most ITK classes.
Abstraction of the Events used to communicating among filters and with GUIs.
A Command subclass that calls a pointer to a C function.
void(T::*)(const EventObject &) TMemberFunctionPointer
void(T::*)(Object *, const EventObject &) TMemberFunctionPointer