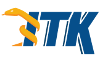 |
ITK
5.1.0
Insight Toolkit
|
Go to the documentation of this file.
28 #ifndef itkConceptChecking_h
29 #define itkConceptChecking_h
36 #ifndef ITK_CONCEPT_NO_CHECKING
37 # if defined(_MSC_VER) && !defined(__ICL)
38 # define ITK_CONCEPT_IMPLEMENTATION_VTABLE
42 # define ITK_CONCEPT_IMPLEMENTATION_STANDARD
47 #if defined(ITK_CONCEPT_IMPLEMENTATION_STANDARD)
59 # define itkConceptConstraintsMacro() \
60 template <void (Constraints::*)()> \
63 using EnforcerInstantiation = Enforcer<&Constraints::constraints>;
64 # define itkConceptMacro(name, concept) \
67 name = sizeof concept \
70 #elif defined(ITK_CONCEPT_IMPLEMENTATION_VTABLE)
77 # define itkConceptConstraintsMacro() \
78 virtual void Enforcer() { &Constraints::constraints; }
79 # define itkConceptMacro(name, concept) \
82 name = sizeof concept \
86 #elif defined(ITK_CONCEPT_IMPLEMENTATION_CALL)
89 # define itkConceptConstraintsMacro()
90 # define itkConceptMacro(name, concept) \
99 # define itkConceptConstraintsMacro()
100 # define itkConceptMacro(name, concept) \
124 template <
typename T>
130 template <
unsigned int>
142 template <
typename T>
151 template <
typename T>
162 template <
typename T>
180 template <
typename T>
213 template <
typename T1,
typename T2>
221 auto b = static_cast<T2>(
a);
232 template <
typename T>
259 template <
typename T1,
typename T2 = T1>
281 template <
typename T1,
typename T2 = T1>
303 template <
typename T1,
typename T2 = T1>
327 template <
typename T1,
typename T2 = T1>
355 template <
typename T1,
typename T2 = T1,
typename T3 = T1>
363 a = static_cast<T3>(
b +
c);
364 a = static_cast<T3>(
b -
c);
372 a = static_cast<T3>(d +
e);
373 a = static_cast<T3>(d -
e);
387 template <
typename T1,
typename T2 = T1>
416 template <
typename T1,
typename T2 = T1,
typename T3 = T1>
424 a = static_cast<T3>(
b *
c);
432 a = static_cast<T3>(d *
e);
443 template <
typename T1,
typename T2 = T1>
470 template <
typename T1,
typename T2 = T1,
typename T3 = T1>
478 a = static_cast<T3>(
b /
c);
486 a = static_cast<T3>(d /
e);
499 template <
typename T1,
typename T2 = T1>
528 template <
typename T1,
typename T2 = T1,
typename T3 = T1>
536 a = static_cast<T3>(
b &
c);
537 a = static_cast<T3>(
b |
c);
538 a = static_cast<T3>(
b ^
c);
539 a &= static_cast<T3>(
c);
540 a |= static_cast<T3>(
c);
541 a ^= static_cast<T3>(
c);
549 a = static_cast<T3>(d &
e);
550 a = static_cast<T3>(d |
e);
551 a = static_cast<T3>(d ^
e);
552 a &= static_cast<T3>(
e);
553 a |= static_cast<T3>(
e);
554 a ^= static_cast<T3>(
e);
566 template <
typename T1,
typename T2 = T1,
typename T3 = T1>
574 a = static_cast<T3>(
b[
c]);
582 a = static_cast<T3>(d[
e]);
594 template <
typename T>
612 template <
typename T>
633 template <
typename T>
651 template <
typename T>
674 template <
typename T1,
typename T2>
691 template <
unsigned int D1,
unsigned int D2>
711 template <
typename T>
749 template <
typename T>
768 template <
typename T>
785 template <
typename T>
804 template <
typename T1,
typename T2>
821 template <
unsigned int D1,
unsigned int D2>
845 template <
unsigned int D1,
unsigned int D2>
873 template <
typename T>
897 template <
typename T>
921 template <
typename T>
944 template <
typename T>
949 static constexpr
bool IsExact = std::numeric_limits<typename NumericTraits<T>::ValueType>::is_exact;
971 template <
typename T>
976 static constexpr
bool IsExact = std::numeric_limits<typename NumericTraits<T>::ValueType>::is_exact;
itkConceptConstraintsMacro()
static constexpr bool Unsigned
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
static bool IsPositive(T val)
itkConceptConstraintsMacro()
Detail::UniqueType_unsigned_int< D2 > DT2
Detail::UniqueType_bool< false > FalseT
static constexpr bool IsExact
void const_constraints(const T1 &d, const T2 &e)
Detail::UniqueType_bool< false > FalseT
static constexpr bool Integral
itkConceptConstraintsMacro()
static constexpr T NonpositiveMin()
itkConceptConstraintsMacro()
Detail::UniqueType_bool< false > FalseT
itkConceptConstraintsMacro()
void const_constraints(const T &a)
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
void const_constraints(const T1 &d, const T2 &e)
Traits for a pixel that define the dimension and component type.
itkConceptConstraintsMacro()
Detail::UniqueType_bool< true > TrueT
void const_constraints(const T1 &d)
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
void const_constraints(const T1 &d)
itkConceptConstraintsMacro()
void const_constraints(const T &b)
static constexpr bool IsSigned
itkConceptConstraintsMacro()
Detail::UniqueType_bool< true > TrueT
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
void const_constraints(const T1 &d, const T2 &e)
void const_constraints(const T1 &d)
void const_constraints(const T1 &d, const T2 &e)
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
void f(Type3, int=0, int=0)
itkConceptConstraintsMacro()
Define additional traits for native types such as int or float.
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
Detail::UniqueType_bool< true > TrueT
static bool IsNonnegative(T val)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
itkConceptConstraintsMacro()
void const_constraints(const T1 &d, const T2 &e)
static bool IsNonpositive(T val)
static constexpr bool Integral
itkConceptConstraintsMacro()
Detail::UniqueType_bool< true > TrueT
static constexpr bool IsExact
static constexpr double e
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
void RequireBooleanExpression(const T &t)
static constexpr bool Integral
static bool IsNegative(T val)
static constexpr bool NonIntegral
itkConceptConstraintsMacro()
void IgnoreUnusedVariable(T)