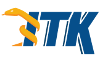 |
ITK
5.1.0
Insight Toolkit
|
Go to the documentation of this file.
19 #ifndef itkImageBufferRange_h
20 #define itkImageBufferRange_h
26 #include <type_traits>
37 namespace Experimental
74 template <
typename TImage>
88 std::is_same<PixelType, InternalPixelType>::value &&
89 std::is_same<typename TImage::AccessorType, DefaultPixelAccessor<PixelType>>::value &&
90 std::is_same<AccessorFunctorType, DefaultPixelAccessorFunctor<typename std::remove_const<TImage>::type>>::value;
99 typename std::conditional<SupportsDirectPixelAccess, EmptyAccessorFunctor, AccessorFunctorType>::type;
108 template <
bool VIsConst,
typename TDummy =
void>
114 template <
typename TDummy>
136 : m_InternalPixel{ internalPixel }
137 , m_AccessorFunctor(accessorFunctor)
142 : m_InternalPixel{ pixelProxy.m_InternalPixel }
143 , m_AccessorFunctor{ pixelProxy.m_AccessorFunctor }
147 operator PixelType() const ITK_NOEXCEPT {
return m_AccessorFunctor.Get(m_InternalPixel); }
153 template <
typename TDummy>
177 : m_InternalPixel{ internalPixel }
178 , m_AccessorFunctor(accessorFunctor)
182 operator PixelType() const ITK_NOEXCEPT {
return m_AccessorFunctor.Get(m_InternalPixel); }
188 m_AccessorFunctor.Set(m_InternalPixel, pixelValue);
207 const auto lhsPixelValue = lhs.m_AccessorFunctor.Get(lhs.m_InternalPixel);
208 const auto rhsPixelValue = rhs.m_AccessorFunctor.Get(rhs.m_InternalPixel);
211 lhs.m_AccessorFunctor.Set(lhs.m_InternalPixel, rhsPixelValue);
212 rhs.m_AccessorFunctor.Set(rhs.m_InternalPixel, lhsPixelValue);
229 template <
bool VIsConst>
247 typename std::conditional<IsImageTypeConst, const InternalPixelType, InternalPixelType>::type;
250 using QualifiedPixelType =
typename std::conditional<IsImageTypeConst, const PixelType, PixelType>::type;
298 typename std::conditional<SupportsDirectPixelAccess, QualifiedPixelType &, PixelProxy<IsImageTypeConst>>::type;
332 using PixelWrapper =
typename std::conditional<SupportsDirectPixelAccess, PixelReferenceWrapper, reference>::type;
390 return lhs.m_InternalPixelPointer == rhs.m_InternalPixelPointer;
399 return !(lhs == rhs);
407 return lhs.m_InternalPixelPointer < rhs.m_InternalPixelPointer;
460 return lhs.m_InternalPixelPointer - rhs.m_InternalPixelPointer;
500 typename std::conditional<IsImageTypeConst, const InternalPixelType, InternalPixelType>::type;
515 result.SetPixelAccessor(
m_Image.GetPixelAccessor());
516 result.SetBegin(
m_Image.ImageType::GetBufferPointer());
538 template <
bool VIsConst>
562 typename std::conditional<UsingPointerAsIterator, const InternalPixelType *, QualifiedIterator<true>>::type;
564 conditional<UsingPointerAsIterator, QualifiedInternalPixelType *, QualifiedIterator<IsImageTypeConst>>::type;
584 ,
m_NumberOfPixels{ image.ImageType::GetBufferedRegion().GetNumberOfPixels() }
611 return this->
begin();
672 assert(n < this->
size());
673 assert(n <= static_cast<std::size_t>(std::numeric_limits<std::ptrdiff_t>::max()));
676 return this->
begin()[static_cast<std::ptrdiff_t>(n)];
684 template <
typename TImage>
685 ImageBufferRange<TImage>
688 if (image ==
nullptr)
PixelProxy(const PixelProxy< false > &pixelProxy) noexcept
reference operator[](const difference_type n) const noexcept
QualifiedIterator & operator=(const QualifiedIterator &) noexcept=default
typename std::conditional< SupportsDirectPixelAccess, QualifiedPixelType &, PixelProxy< IsImageTypeConst > >::type reference
QualifiedInternalPixelType * m_ImageBufferPointer
typename std::conditional< IsImageTypeConst, const PixelType, PixelType >::type QualifiedPixelType
PixelProxy(InternalPixelType &internalPixel, const AccessorFunctorType &accessorFunctor) noexcept
InternalPixelType & m_InternalPixel
friend QualifiedIterator operator-(QualifiedIterator it, const difference_type n) noexcept
PixelReferenceWrapper(QualifiedPixelType &pixel, EmptyAccessorFunctor) noexcept
iterator begin() const noexcept
std::vcl_size_t size() const noexcept
typename std::conditional< SupportsDirectPixelAccess, EmptyAccessorFunctor, AccessorFunctorType >::type OptionalAccessorFunctorType
friend QualifiedIterator operator+(const difference_type n, QualifiedIterator it) noexcept
typename std::conditional< IsImageTypeConst, const InternalPixelType, InternalPixelType >::type QualifiedInternalPixelType
const_iterator cend() const noexcept
friend bool operator!=(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
QualifiedPixelType & m_Pixel
friend bool operator==(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
constexpr static bool UsingPointerAsIterator
typename TImage::InternalPixelType InternalPixelType
QualifiedIterator & operator++() noexcept
ImageBufferRange()=default
reverse_iterator rbegin() const noexcept
OptionalAccessorFunctorType m_OptionalAccessorFunctor
PixelProxy(const InternalPixelType &internalPixel, const AccessorFunctorType &accessorFunctor) noexcept
QualifiedIterator(const QualifiedIterator< false > &arg) noexcept
PixelProxy & operator=(const PixelType &pixelValue) noexcept
const_iterator cbegin() const noexcept
std::ptrdiff_t difference_type
SizeValueType m_NumberOfPixels
friend QualifiedIterator & operator+=(QualifiedIterator &it, const difference_type n) noexcept
typename TImage::PixelType PixelType
friend difference_type operator-(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
QualifiedInternalPixelType * m_InternalPixelPointer
friend bool operator>(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
friend bool operator>=(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
typename std::conditional< UsingPointerAsIterator, QualifiedInternalPixelType *, QualifiedIterator< IsImageTypeConst > >::type iterator
friend bool operator<(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
std::reverse_iterator< iterator > reverse_iterator
ImageBufferRange(ImageType &image)
const AccessorFunctorType m_AccessorFunctor
PixelProxy & operator=(const PixelProxy &pixelProxy) noexcept
QualifiedPixelType * pointer
AccessorFunctorInitializer(ImageType &image) noexcept
static constexpr bool IsImageTypeConst
friend void swap(PixelProxy lhs, PixelProxy rhs) noexcept
std::random_access_iterator_tag iterator_category
OptionalAccessorFunctorType m_OptionalAccessorFunctor
friend QualifiedIterator & operator-=(QualifiedIterator &it, const difference_type n) noexcept
QualifiedIterator(const OptionalAccessorFunctorType &accessorFunctor, QualifiedInternalPixelType *const internalPixelPointer) noexcept
reverse_iterator rend() const noexcept
const AccessorFunctorType m_AccessorFunctor
typename std::conditional< VIsConst, const ImageType, ImageType >::type QualifiedImageType
typename std::conditional< IsImageTypeConst, const InternalPixelType, InternalPixelType >::type QualifiedInternalPixelType
const InternalPixelType & m_InternalPixel
QualifiedInternalPixelType * m_InternalPixelPointer
QualifiedIterator & operator--() noexcept
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
const_reverse_iterator crbegin() const noexcept
constexpr static bool SupportsDirectPixelAccess
QualifiedIterator operator++(int) noexcept
iterator end() const noexcept
friend QualifiedIterator operator+(QualifiedIterator it, const difference_type n) noexcept
static constexpr bool IsImageTypeConst
typename std::conditional< UsingPointerAsIterator, const InternalPixelType *, QualifiedIterator< true > >::type const_iterator
std::reverse_iterator< const_iterator > const_reverse_iterator
ImageBufferRange< TImage > MakeImageBufferRange(TImage *const image)
typename TImage::AccessorFunctorType AccessorFunctorType
QualifiedIterator< false >::reference operator[](const std::vcl_size_t n) const noexcept
reference operator*() const noexcept
QualifiedIterator operator--(int) noexcept
friend bool operator<=(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
IteratorInitializer(OptionalAccessorFunctorType optionalAccessorFunctor, QualifiedInternalPixelType *internalPixelPointer) noexcept
const_reverse_iterator crend() const noexcept
OptionalAccessorFunctorType m_OptionalAccessorFunctor
unsigned long SizeValueType
bool empty() const noexcept
QualifiedIterator()=default