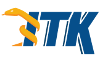 |
ITK
5.1.0
Insight Toolkit
|
Go to the documentation of this file.
70 template <
typename TLabelObject>
74 ITK_DISALLOW_COPY_AND_ASSIGN(
LabelMap);
101 static constexpr
unsigned int ImageDimension = LabelObjectType::ImageDimension;
141 Initialize()
override;
145 Allocate(
bool initialize =
false)
override;
148 Graft(
const Self * imgData);
158 GetLabelObject(
const LabelType & label)
const;
236 GetLabelObject(
const IndexType & idx)
const;
274 GetNumberOfLabelObjects()
const;
286 GetLabelObjects()
const;
291 itkGetConstMacro(BackgroundValue,
LabelType);
300 PrintLabelObjects(std::ostream & os)
const;
305 this->PrintLabelObjects(std::cerr);
326 m_Begin = lm->m_LabelObjectContainer.begin();
327 m_End = lm->m_LabelObjectContainer.end();
328 m_Iterator = m_Begin;
350 return m_Iterator->second;
356 return m_Iterator->first;
383 return !(*
this == iter);
389 m_Iterator = m_Begin;
395 return m_Iterator == m_End;
417 m_Begin = lm->m_LabelObjectContainer.begin();
418 m_End = lm->m_LabelObjectContainer.end();
419 m_Iterator = m_Begin;
441 return m_Iterator->second;
447 return m_Iterator->first;
474 return !(*
this == iter);
480 m_Iterator = m_Begin;
486 return m_Iterator == m_End;
502 PrintSelf(std::ostream & os,
Indent indent)
const override;
505 using Superclass::Graft;
524 #ifndef ITK_MANUAL_INSTANTIATION
525 # include "itkLabelMap.hxx"
typename LabelObjectType::Pointer LabelObjectPointerType
A forward iterator over the LabelObjects of a LabelMap.
typename Superclass::SizeValueType SizeValueType
ImageBaseType::DirectionType DirectionType
bool operator==(const ConstIterator &iter) const
bool operator!=(const ConstIterator &iter) const
InternalIteratorType m_Begin
const LabelType & GetLabel() const
bool operator!=(const Iterator &iter) const
std::vector< LabelObjectPointerType > LabelObjectVectorType
typename LabelObjectType::LabelType LabelType
Base class for templated image classes.
A forward iterator over the LabelObjects of a LabelMap.
ImageBaseType::PointType PointType
typename Superclass::OffsetType OffsetType
ImageBaseType::SizeType SizeType
InternalIteratorType m_End
InternalIteratorType m_Begin
Control indentation during Print() invocation.
typename Superclass::SpacingType SpacingType
typename LabelObjectContainerType::const_iterator LabelObjectContainerConstIterator
LabelType m_BackgroundValue
ConstIterator operator++(int)
Templated n-dimensional image to store labeled objects.
typename Superclass::RegionType RegionType
typename LabelObjectContainerType::iterator LabelObjectContainerIterator
ImageBaseType::IndexType IndexType
typename Superclass::DirectionType DirectionType
InternalIteratorType m_End
ConstIterator(const ConstIterator &iter)
ConstIterator & operator++()
std::map< LabelType, LabelObjectPointerType > LabelObjectContainerType
typename std::map< LabelType, LabelObjectPointerType >::const_iterator InternalIteratorType
ImageBaseType::RegionType RegionType
void PrintLabelObjects() const
const LabelType & GetLabel() const
Iterator & operator=(const Iterator &iter)
std::vector< LabelType > LabelVectorType
TLabelObject LabelObjectType
typename Superclass::PointType PointType
typename Superclass::SizeType SizeType
InternalIteratorType m_Iterator
typename std::map< LabelType, LabelObjectPointerType >::iterator InternalIteratorType
const LabelObjectType * GetLabelObject() const
Implements a weak reference to an object.
Iterator(const Iterator &iter)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
ConstIterator(const Self *lm)
signed long OffsetValueType
LabelObjectContainerType m_LabelObjectContainer
InternalIteratorType m_Iterator
Base class for most ITK classes.
ConstIterator & operator=(const ConstIterator &iter)
bool operator==(const Iterator &iter) const
typename Superclass::OffsetValueType OffsetValueType
typename Superclass::IndexType IndexType
unsigned long SizeValueType
LabelObjectType * GetLabelObject()
Base class for all data objects in ITK.