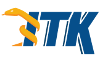 |
ITK
5.1.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkLabelObjectAccessors_h
19 #define itkLabelObjectAccessors_h
37 template <
typename TLabelObject>
47 return labelObject->GetLabel();
51 template <
typename TLabelObject>
61 return labelObject->GetNumberOfLines();
65 template <
typename TLabelObject,
typename TAttributeAccessor>
84 template <
typename TLabelObject,
typename TAttributeAccessor>
TLabelObject LabelObjectType
bool operator()(const LabelObjectType *a, const LabelObjectType *b) const
TLabelObject LabelObjectType
AttributeValueType operator()(const LabelObjectType *labelObject) const
LabelObjectReverseComparator()=default
TAttributeAccessor AttributeAccessorType
AttributeAccessorType m_Accessor
LabelObjectReverseComparator(LabelObjectReverseComparator const &from)
LabelObjectComparator()=default
TLabelObject LabelObjectType
AttributeAccessorType m_Accessor
AttributeValueType operator()(const LabelObjectType *labelObject) const
TLabelObject LabelObjectType
LabelObjectComparator(LabelObjectComparator const &from)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
TAttributeAccessor AttributeAccessorType
bool operator()(const LabelObjectType *a, const LabelObjectType *b) const
typename LabelObjectType::LabelType AttributeValueType