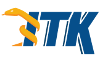 |
ITK
5.1.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkShapeLabelObjectAccessors_h
19 #define itkShapeLabelObjectAccessors_h
38 template <
typename TLabelObject>
48 return labelObject->GetNumberOfPixels();
52 template <
typename TLabelObject>
62 return labelObject->GetBoundingBox();
66 template <
typename TLabelObject>
76 return labelObject->GetPhysicalSize();
80 template <
typename TLabelObject>
90 return labelObject->GetNumberOfPixelsOnBorder();
94 template <
typename TLabelObject>
104 return labelObject->GetPerimeterOnBorder();
108 template <
typename TLabelObject>
118 return labelObject->GetCentroid();
122 template <
typename TLabelObject>
132 return labelObject->GetFeretDiameter();
136 template <
typename TLabelObject>
146 return labelObject->GetPrincipalMoments();
150 template <
typename TLabelObject>
160 return labelObject->GetPrincipalAxes();
164 template <
typename TLabelObject>
174 return labelObject->GetElongation();
178 template <
typename TLabelObject>
188 return labelObject->GetPerimeter();
192 template <
typename TLabelObject>
202 return labelObject->GetRoundness();
206 template <
typename TLabelObject>
216 return labelObject->GetEquivalentSphericalRadius();
220 template <
typename TLabelObject>
230 return labelObject->GetEquivalentSphericalPerimeter();
234 template <
typename TLabelObject>
244 return labelObject->GetEquivalentEllipsoidDiameter();
248 template <
typename TLabelObject>
258 return labelObject->GetFlatness();
262 template <
typename TLabelObject>
272 return labelObject->GetPerimeterOnBorderRatio();
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
typename LabelObjectType::MatrixType AttributeValueType
TLabelObject LabelObjectType
TLabelObject LabelObjectType
AttributeValueType operator()(const LabelObjectType *labelObject) const
double AttributeValueType
ImageBaseType::SpacingType VectorType
TLabelObject LabelObjectType
TLabelObject LabelObjectType
typename LabelObjectType::VectorType AttributeValueType
SizeValueType AttributeValueType
TLabelObject LabelObjectType
AttributeValueType operator()(const LabelObjectType *labelObject) const
double AttributeValueType
SizeValueType AttributeValueType
double AttributeValueType
typename LabelObjectType::CentroidType AttributeValueType
double AttributeValueType
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
ImageBaseType::RegionType RegionType
TLabelObject LabelObjectType
double AttributeValueType
AttributeValueType operator()(const LabelObjectType *labelObject) const
TLabelObject LabelObjectType
TLabelObject LabelObjectType
TLabelObject LabelObjectType
TLabelObject LabelObjectType
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
TLabelObject LabelObjectType
double AttributeValueType
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
double AttributeValueType
AttributeValueType operator()(const LabelObjectType *labelObject) const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
double AttributeValueType
TLabelObject LabelObjectType
double AttributeValueType
AttributeValueType operator()(const LabelObjectType *labelObject) const
AttributeValueType operator()(const LabelObjectType *labelObject) const
TLabelObject LabelObjectType
typename LabelObjectType::RegionType AttributeValueType
TLabelObject LabelObjectType
TLabelObject LabelObjectType
typename LabelObjectType::VectorType AttributeValueType
TLabelObject LabelObjectType
unsigned long SizeValueType
double AttributeValueType
TLabelObject LabelObjectType
AttributeValueType operator()(const LabelObjectType *labelObject) const