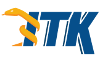 |
ITK
5.1.0
Insight Toolkit
|
Go to the documentation of this file.
19 #ifndef itkSmartPointer_h
20 #define itkSmartPointer_h
24 #include <type_traits>
25 #include "itkConfigure.h"
50 template <
typename TObjectType>
76 template <typename T, typename = typename EnableIfConvertible<T>::type>
87 p.m_Pointer =
nullptr;
91 template <typename T, typename = typename EnableIfConvertible<T>::type>
95 p.m_Pointer =
nullptr;
118 explicit operator bool() const noexcept {
return m_Pointer !=
nullptr; }
181 (*m_Pointer).Print(os);
187 #if !defined(ITK_LEGACY_REMOVE)
200 other.m_Pointer = tmp;
207 template <
typename T>
231 template <
class T,
class TU>
235 return (l.GetPointer() == r.GetPointer());
241 return (l.GetPointer() ==
nullptr);
247 return (
nullptr == r.GetPointer());
252 template <
class T,
class TU>
256 return (l.GetPointer() != r.GetPointer());
262 return (l.GetPointer() !=
nullptr);
268 return (
nullptr != r.GetPointer());
274 template <
class T,
class TU>
278 return (l.GetPointer() < r.GetPointer());
282 template <
class T,
class TU>
286 return (l.GetPointer() > r.GetPointer());
290 template <
class T,
class TU>
294 return (l.GetPointer() <= r.GetPointer());
298 template <
class T,
class TU>
302 return (l.GetPointer() >= r.GetPointer());
305 template <
typename T>
313 template <
typename T>
void Swap(SmartPointer &other) noexcept
SmartPointer(const SmartPointer< T > &p) noexcept
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
typename std::enable_if< std::is_convertible< T *, const Self * >::value > EnableIfConvertible
bool operator<=(const Index< VDimension > &one, const Index< VDimension > &two)
SmartPointer(ObjectType *p) noexcept
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
void swap(Array< T > &a, Array< T > &b)
SmartPointer(SmartPointer< ObjectType > &&p) noexcept
SmartPointer & operator=(SmartPointer r) noexcept
Implements transparent reference counting.
SmartPointer(const SmartPointer &p) noexcept
ObjectType * Print(std::ostream &os) const
constexpr SmartPointer(std::nullptr_t p) noexcept
ObjectType * GetPointer() const noexcept
void UnRegister() noexcept
ObjectType & operator*() const noexcept
bool operator>=(const Index< VDimension > &one, const Index< VDimension > &two)
ObjectType * operator->() const noexcept
bool operator>(const Index< VDimension > &one, const Index< VDimension > &two)
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
bool IsNull() const noexcept
SmartPointer(SmartPointer< T > &&p) noexcept
bool operator!=(const Index< VDimension > &one, const Index< VDimension > &two)
SmartPointer & operator=(std::nullptr_t) noexcept
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
bool IsNotNull() const noexcept
constexpr SmartPointer() noexcept