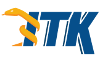 |
ITK
5.1.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkSpatialObjectPoint_h
19 #define itkSpatialObjectPoint_h
24 #include "vnl/vnl_vector_fixed.h"
40 template <
unsigned int TDimension,
class TSpatialObjectPo
intType>
41 class PointBasedSpatialObject;
43 template <
unsigned int TPo
intDimension = 3>
56 return "SpatialObjectPoint";
85 m_PositionInObjectSpace = newPositionInObjectSpace;
88 template <
typename... TCoordinate>
92 static_assert((1 +
sizeof...(otherCoordinate)) == TPointDimension,
93 "The number of coordinates must be equal to the dimensionality!");
94 const double coordinates[] = { firstCoordinate, static_cast<double>(otherCoordinate)... };
95 m_PositionInObjectSpace = coordinates;
102 return m_PositionInObjectSpace;
108 m_SpatialObject = so;
114 return m_SpatialObject;
120 SetPositionInWorldSpace(
const PointType & point);
125 GetPositionInWorldSpace()
const;
146 SetColor(
double r,
double g,
double b,
double a = 1);
158 return m_Color.GetRed();
171 return m_Color.GetGreen();
184 return m_Color.GetBlue();
197 return m_Color.GetAlpha();
204 this->PrintSelf(os, 3);
210 PrintSelf(std::ostream & os,
Indent indent)
const;
229 #ifndef ITK_MANUAL_INSTANTIATION
230 # include "itkSpatialObjectPoint.hxx"
233 #endif // itkSpatialObjectPoint_h
void Print(std::ostream &os) const
void SetPositionInObjectSpace(const double firstCoordinate, const TCoordinate... otherCoordinate)
void SetPositionInObjectSpace(const PointType &newPositionInObjectSpace)
ImageBaseType::PointType PointType
void SetSpatialObject(SpatialObjectType *so)
Control indentation during Print() invocation.
ColorType GetColor() const
WeakPointer< SpatialObjectType > m_SpatialObject
void SetColor(ColorType color)
Implementation of the composite pattern.
virtual const char * GetNameOfClass() const
SpatialObjectType * GetSpatialObject() const
Implements a weak reference to an object.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
PointType m_PositionInObjectSpace
const PointType & GetPositionInObjectSpace() const
vnl_vector< double > VectorType
Point used for spatial objets.