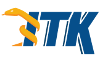 |
ITK
5.1.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkVectorContainer_h
19 #define itkVectorContainer_h
47 template <
typename TElementIdentifier,
typename TElement>
50 ,
private std::vector<TElement>
90 template <
typename TInputIterator>
119 const STLContainerType &
125 using STLContainerType::begin;
126 using STLContainerType::end;
127 using STLContainerType::rbegin;
128 using STLContainerType::rend;
129 using STLContainerType::cbegin;
130 using STLContainerType::cend;
131 using STLContainerType::crbegin;
132 using STLContainerType::crend;
134 using STLContainerType::size;
135 using STLContainerType::max_size;
136 using STLContainerType::resize;
137 using STLContainerType::capacity;
138 using STLContainerType::empty;
139 using STLContainerType::reserve;
140 using STLContainerType::shrink_to_fit;
142 using STLContainerType::operator[];
143 using STLContainerType::at;
144 using STLContainerType::front;
145 using STLContainerType::back;
147 using STLContainerType::assign;
148 using STLContainerType::push_back;
149 using STLContainerType::pop_back;
150 using STLContainerType::insert;
151 using STLContainerType::erase;
153 using STLContainerType::clear;
155 using STLContainerType::get_allocator;
157 using typename STLContainerType::reference;
158 using typename STLContainerType::const_reference;
159 using typename STLContainerType::iterator;
160 using typename STLContainerType::const_iterator;
163 using typename STLContainerType::difference_type;
164 using typename STLContainerType::value_type;
165 using typename STLContainerType::allocator_type;
166 using typename STLContainerType::pointer;
167 using typename STLContainerType::const_pointer;
168 using typename STLContainerType::reverse_iterator;
169 using typename STLContainerType::const_reverse_iterator;
172 friend class Iterator;
173 friend class ConstIterator;
186 using pointer =
typename VectorIterator::pointer;
236 return static_cast<difference_type>(this->m_Pos) - static_cast<difference_type>(r.
m_Pos);
242 return m_Iter == r.
m_Iter;
247 return m_Iter != r.
m_Iter;
252 return m_Iter == r.
m_Iter;
257 return m_Iter != r.
m_Iter;
262 return (this->
operator-(r)) < 0;
293 return static_cast<ElementIdentifier>(m_Pos);
320 using pointer =
typename VectorConstIterator::pointer;
321 using reference =
typename VectorConstIterator::reference;
384 return static_cast<difference_type>(m_Pos) - static_cast<difference_type>(r.
m_Pos);
390 return m_Iter == r.
m_Iter;
395 return m_Iter != r.
m_Iter;
400 return m_Iter == r.
m_Iter;
405 return m_Iter != r.
m_Iter;
410 return (this->
operator-(r) < 0);
434 return static_cast<ElementIdentifier>(m_Pos);
585 #ifndef ITK_MANUAL_INSTANTIATION
586 # include "itkVectorContainer.hxx"
ConstIterator operator++(int)
typename VectorType::const_iterator VectorConstIterator
typename VectorIterator::reference reference
VectorContainer(size_type n)
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
bool operator<=(const Index< VDimension > &one, const Index< VDimension > &two)
Represent a n-dimensional size (bounds) of a n-dimensional image.
typename VectorType::size_type size_type
VectorContainer(TInputIterator first, TInputIterator last)
typename VectorConstIterator::value_type value_type
difference_type operator-(const Iterator &r) const
typename VectorConstIterator::reference reference
VectorType STLContainerType
ImageBaseType::SpacingType VectorType
Iterator & operator+=(difference_type n)
void swap(Array< T > &a, Array< T > &b)
ConstIterator & operator--()
typename VectorType::iterator VectorIterator
typename VectorIterator::difference_type difference_type
ElementIdentifier Index() const
bool operator==(const Iterator &r) const
TElementIdentifier ElementIdentifier
ConstIterator & operator+=(difference_type n)
bool operator!=(const ConstIterator &r) const
const STLContainerType & CastToSTLConstContainer() const noexcept
ConstIterator & operator*()
const_reference Value() const
bool operator>(const Iterator &r) const
Light weight base class for most itk classes.
typename VectorConstIterator::iterator_category iterator_category
bool operator>=(const Iterator &r) const
bool operator==(const ConstIterator &r) const
ConstIterator & operator=(const Iterator &r)
Iterator(size_type d, const VectorIterator &i)
bool operator==(const Iterator &r) const
typename VectorIterator::iterator_category iterator_category
ConstIterator(size_type d, const VectorConstIterator &i)
ElementIdentifier Index() const
bool operator!=(const Iterator &r) const
ConstIterator * operator->()
Iterator(const Iterator &r)
VectorConstIterator m_Iter
bool operator>=(const ConstIterator &r) const
typename VectorIterator::value_type value_type
VectorContainer(const Self &r)
bool operator==(const ConstIterator &r) const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename VectorConstIterator::difference_type difference_type
std::vector< Element > VectorType
difference_type operator-(const ConstIterator &r) const
Base class for most ITK classes.
bool operator>(const ConstIterator &r) const
STLContainerType & CastToSTLContainer() noexcept
ConstIterator & operator++()
ConstIterator(const Iterator &r)
ConstIterator operator--(int)
bool operator!=(const ConstIterator &r) const
typename VectorIterator::pointer pointer
bool operator!=(const Iterator &r) const
typename VectorConstIterator::pointer pointer
Define a front-end to the STL "vector" container that conforms to the IndexedContainerInterface.
VectorContainer(size_type n, const Element &x)