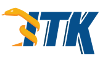 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkBitwiseOpsFunctors_h
19 #define itkBitwiseOpsFunctors_h
33 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
34 class ITK_TEMPLATE_EXPORT
AND
49 return !(*
this != other);
55 return static_cast<TOutput>(A & B);
64 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
65 class ITK_TEMPLATE_EXPORT
OR
80 return !(*
this != other);
86 return static_cast<TOutput>(A | B);
95 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
96 class ITK_TEMPLATE_EXPORT
XOR
111 return !(*
this != other);
117 return static_cast<TOutput>(A ^ B);
126 template <
class TInput,
class TOutput>
142 return !(*
this != other);
148 return static_cast<TOutput>(~A);
bool operator==(const OR &other) const
bool operator!=(const XOR &) const
bool operator==(const BitwiseNot &other) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const
bool operator!=(const BitwiseNot &) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const
bool operator!=(const AND &) const
Performs the C++ unary bitwise NOT operator.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
TOutput operator()(const TInput &A) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const
bool operator==(const AND &other) const
bool operator==(const XOR &other) const
bool operator!=(const OR &) const