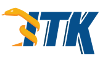 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkCellInterface_h
19 #define itkCellInterface_h
32 #define itkCellVisitMacro(TopologyId) \
33 static constexpr CellGeometryEnum GetTopologyId() { return TopologyId; } \
34 virtual void Accept(CellIdentifier cellid, typename CellInterface<PixelType, CellTraits>::MultiVisitor * mv) \
37 typename CellInterfaceVisitor<PixelType, CellTraits>::Pointer v = mv->GetVisitor(TopologyId); \
40 v->VisitFromCell(cellid, this); \
46 #define itkCellCommonTypedefs(celltype) \
47 using Self = celltype; \
48 using ConstSelfAutoPointer = AutoPointer<const Self>; \
49 using SelfAutoPointer = AutoPointer<Self>; \
50 using RawPointer = Self *; \
51 using ConstRawPointer = const Self *
55 #define itkCellInheritedTypedefs(superclassArg) \
56 using Superclass = superclassArg; \
57 using PixelType = typename Superclass::PixelType; \
58 using CellType = typename Superclass::CellType; \
59 using CellAutoPointer = typename Superclass::CellAutoPointer; \
60 using CellConstAutoPointer = typename Superclass::CellConstAutoPointer; \
61 using CellRawPointer = typename Superclass::CellRawPointer; \
62 using CellConstRawPointer = typename Superclass::CellConstRawPointer; \
63 using CellTraits = typename Superclass::CellTraits; \
64 using CoordRepType = typename Superclass::CoordRepType; \
65 using InterpolationWeightType = typename Superclass::InterpolationWeightType; \
66 using PointIdentifier = typename Superclass::PointIdentifier; \
67 using PointIdIterator = typename Superclass::PointIdIterator; \
68 using PointIdConstIterator = typename Superclass::PointIdConstIterator; \
69 using CellIdentifier = typename Superclass::CellIdentifier; \
70 using CellFeatureIdentifier = typename Superclass::CellFeatureIdentifier; \
71 using CellFeatureCount = typename Superclass::CellFeatureIdentifier; \
72 using PointType = typename Superclass::PointType; \
73 using VectorType = typename Superclass::VectorType; \
74 using PointsContainer = typename Superclass::PointsContainer; \
75 using UsingCellsContainer = typename Superclass::UsingCellsContainer; \
76 using ParametricCoordArrayType = typename Superclass::ParametricCoordArrayType; \
77 using ShapeFunctionsArrayType = typename Superclass::ShapeFunctionsArrayType; \
78 static constexpr unsigned int PointDimension = Superclass::PointDimension
95 template <
typename TPixelType,
typename TCellTraits>
126 static constexpr
unsigned int PointDimension = CellTraits::PointDimension;
190 return m_Visitors[static_cast<int>(
id)];
194 auto pos = m_UserDefined.find(
id);
195 if (pos != m_UserDefined.end())
197 return (*pos).second;
210 m_Visitors[static_cast<int>(
id)] = v;
234 virtual ::itk::CommonEnums::CellGeometry
244 GetDimension()
const = 0;
248 GetInterpolationOrder()
const;
252 GetNumberOfPoints()
const = 0;
256 GetNumberOfBoundaryFeatures(
int dimension)
const = 0;
293 PointIdsBegin()
const = 0;
302 PointIdsEnd()
const = 0;
307 GetPointIdsContainer()
const;
423 IsExplicitBoundary();
430 AddUsingCell(CellIdentifier cellId);
436 RemoveUsingCell(CellIdentifier cellId);
444 IsUsingCell(CellIdentifier cellId);
450 GetNumberOfUsingCells();
452 #if !defined(ITK_WRAPPING_PARSER)
456 virtual UsingCellsContainerIterator
462 virtual UsingCellsContainerIterator
478 #if !defined(ITK_LEGACY_REMOVE)
517 template <
int VPointDimension,
519 typename TInterpolationWeight,
520 typename TPointIdentifier,
521 typename TCellIdentifier,
522 typename TCellFeatureIdentifier,
524 typename TPointsContainer,
525 typename TUsingCellsContainer>
529 static constexpr
unsigned int PointDimension = VPointDimension;
543 #define itkMakeCellTraitsMacro \
544 CellTraitsInfo<Self::PointDimension, \
546 InterpolationWeightType, \
549 CellFeatureIdentifier, \
555 #if !defined(ITK_WRAPPING_PARSER)
556 # ifndef ITK_MANUAL_INSTANTIATION
557 # include "itkCellInterface.hxx"
TPointsContainer PointsContainer
virtual bool GetClosestBoundary(CoordRepType[], bool *, CellAutoPointer &)
typename std::map< CellGeometryEnum, VisitorPointer >::value_type VisitorPointerValueType
std::map< CellGeometryEnum, VisitorPointer > m_UserDefined
typename PointType::VectorType VectorType
NOTE: it should normally be defined in the traits.
typename CellTraits::PointIdentifier PointIdentifier
virtual bool IntersectWithLine(CoordRepType[PointDimension], CoordRepType[PointDimension], CoordRepType, CoordRepType[PointDimension], CoordRepType *, CoordRepType[])
typename CellTraits::UsingCellsContainer UsingCellsContainer
typename VisitorType::Pointer VisitorPointer
Abstract interface for a visitor class that can visit the cells in a Mesh.
A simple utility class to define the cell type inside a mesh type structure definition....
typename CellTraits::InterpolationWeightType InterpolationWeightType
ImageBaseType::SpacingType VectorType
ImageBaseType::PointType PointType
const PointIdentifier * PointIdConstIterator
typename CellTraits::PointsContainer PointsContainer
typename CellTraits::PointType PointType
ConstSelfAutoPointer CellConstAutoPointer
typename CellTraits::CoordRepType CoordRepType
A visitor that can visit different cell types in a mesh. CellInterfaceVisitor instances can be regist...
typename CellTraits::PointIdConstIterator PointIdConstIterator
TCellIdentifier CellIdentifier
PointIdentifier * PointIdIterator
VisitorType * GetVisitor(CellGeometryEnum id)
typename UsingCellsContainer::iterator UsingCellsContainerIterator
Light weight base class for most itk classes.
RawPointer CellRawPointer
CoordRepType * GetBoundingBox(CoordRepType[PointDimension *2])
TUsingCellsContainer UsingCellsContainer
class ITK_TEMPLATE_EXPORT CellInterface
virtual bool EvaluatePosition(CoordRepType *, PointsContainer *, CoordRepType *, CoordRepType[], double *, InterpolationWeightType *)
SelfAutoPointer CellAutoPointer
void UnRegister() noexcept
typename CellTraits::CellIdentifier CellIdentifier
ConstRawPointer CellConstRawPointer
CellFeatureIdentifier CellFeatureCount
An abstract interface for cells.
CoordRepType GetBoundingBoxDiagonalLength2()
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename CellTraits::PointIdIterator PointIdIterator
virtual void EvaluateShapeFunctions(const ParametricCoordArrayType &, ShapeFunctionsArrayType &) const
Array class with size defined at construction time.
TInterpolationWeight InterpolationWeightType
typename CellTraits::CellFeatureIdentifier CellFeatureIdentifier
TCellFeatureIdentifier CellFeatureIdentifier
UsingCellsContainer m_UsingCells
void AddVisitor(VisitorType *v)
TPointIdentifier PointIdentifier
virtual CellGeometryEnum GetCellTopologyId()=0
#define itkCellCommonTypedefs(celltype)
virtual bool IntersectBoundingBoxWithLine(CoordRepType[PointDimension *2], CoordRepType[PointDimension], CoordRepType[PointDimension], CoordRepType[PointDimension], CoordRepType *)