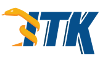 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkChangeLabelImageFilter_h
19 #define itkChangeLabelImageFilter_h
53 template <
typename TInput,
typename TOutput>
76 return !(*
this != other);
82 return m_ChangeMap[original];
86 SetChange(
const TInput & original,
const TOutput & result)
88 m_ChangeMap[original] = result;
94 m_ChangeMap = changeMap;
106 const typename ChangeMapType::const_iterator it = m_ChangeMap.find(A);
107 if (it != m_ChangeMap.end())
119 template <
typename TInputImage,
typename TOutputImage>
124 Functor::ChangeLabel<typename TInputImage::PixelType, typename TOutputImage::PixelType>>
164 #ifdef ITK_USE_CONCEPT_CHECKING
175 PrintSelf(std::ostream & os,
Indent indent)
const override;
179 #ifndef ITK_MANUAL_INSTANTIATION
180 # include "itkChangeLabelImageFilter.hxx"
TOutput operator()(const TInput &A) const
Implements pixel-wise generic operation on one image.
void SetChangeMap(const ChangeMapType &changeMap)
std::map< TInputImage::PixelType, TOutputImage::PixelType > ChangeMapType
Control indentation during Print() invocation.
void SetChange(const TInput &original, const TOutput &result)
Base class for all process objects that output image data.
typename TInputImage::PixelType InputPixelType
bool operator==(const ChangeLabel &other) const
ChangeMapType m_ChangeMap
TOutput GetChange(const TInput &original)
std::map< InputPixelType, OutputPixelType > ChangeMapType
#define itkConceptMacro(name, concept)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
bool operator!=(const ChangeLabel &other) const
typename TOutputImage::PixelType OutputPixelType