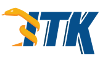 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
48 ITK_DISALLOW_COPY_AND_MOVE(
Command);
112 m_MemberFunction = memberFunction;
119 m_ConstMemberFunction = memberFunction;
126 if (m_MemberFunction)
128 ((*m_This).*(m_MemberFunction))(caller, event);
137 if (m_ConstMemberFunction)
139 ((*m_This).*(m_ConstMemberFunction))(caller, event);
151 , m_MemberFunction(nullptr)
152 , m_ConstMemberFunction(nullptr)
167 template <
typename T>
192 m_MemberFunction = memberFunction;
199 if (m_MemberFunction)
201 ((*m_This).*(m_MemberFunction))(event);
210 if (m_MemberFunction)
212 ((*m_This).*(m_MemberFunction))(event);
223 , m_MemberFunction(nullptr)
238 template <
typename T>
262 m_MemberFunction = memberFunction;
269 if (m_MemberFunction)
271 ((*m_This).*(m_MemberFunction))();
279 if (m_MemberFunction)
281 ((*m_This).*(m_MemberFunction))();
291 , m_MemberFunction(nullptr)
306 template <
typename T>
330 m_MemberFunction = memberFunction;
337 if (m_MemberFunction)
339 ((*m_This).*(m_MemberFunction))();
347 if (m_MemberFunction)
349 ((*m_This).*(m_MemberFunction))();
359 , m_MemberFunction(nullptr)
399 SetClientData(
void * cd);
424 void * m_ClientData{
nullptr };
void(T::*)() TMemberFunctionPointer
A Command subclass that calls a std::function object.
void SetCallbackFunction(T *object, TConstMemberFunctionPointer memberFunction)
A Command subclass that calls a pointer to a member function.
void SetCallbackFunction(const T *object, TMemberFunctionPointer memberFunction)
TMemberFunctionPointer m_MemberFunction
void(T::*)(const Object *, const EventObject &) TConstMemberFunctionPointer
void Execute(Object *, const EventObject &) override
A Command subclass that calls a pointer to a member function.
void(*)(const Object *, const EventObject &, void *) ConstFunctionPointer
void Execute(const Object *caller, const EventObject &event) override
A Command subclass that calls a pointer to a member function.
void Execute(const Object *, const EventObject &) override
void(*)(void *) DeleteDataFunctionPointer
void Execute(Object *, const EventObject &event) override
std::function< void(const EventObject &)> FunctionObjectType
Superclass for callback/observer methods.
void Execute(const Object *, const EventObject &event) override
FunctionObjectType m_FunctionObject
void Execute(Object *caller, const EventObject &event) override
SimpleConstMemberCommand()
class ITK_FORWARD_EXPORT Command
void Execute(const Object *, const EventObject &) override
TMemberFunctionPointer m_MemberFunction
TConstMemberFunctionPointer m_ConstMemberFunction
void Execute(Object *, const EventObject &) override
void SetCallbackFunction(T *object, TMemberFunctionPointer memberFunction)
A Command subclass that calls a pointer to a member function.
void SetCallbackFunction(T *object, TMemberFunctionPointer memberFunction)
TMemberFunctionPointer m_MemberFunction
void(T::*)() const TMemberFunctionPointer
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
void SetCallbackFunction(T *object, TMemberFunctionPointer memberFunction)
void(*)(Object *, const EventObject &, void *) FunctionPointer
TMemberFunctionPointer m_MemberFunction
Base class for most ITK classes.
Abstraction of the Events used to communicating among filters and with GUIs.
A Command subclass that calls a pointer to a C function.
void(T::*)(const EventObject &) TMemberFunctionPointer
void(T::*)(Object *, const EventObject &) TMemberFunctionPointer