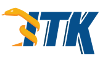 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
20 #ifndef itkFFTWComplexToComplexFFTImageFilter_h
21 # define itkFFTWComplexToComplexFFTImageFilter_h
58 template <
typename TImage>
90 static constexpr
unsigned int ImageDimension = ImageType::ImageDimension;
109 # ifndef ITK_USE_CUFFTW
113 if (m_PlanRigor != value)
119 itkGetConstReferenceMacro(PlanRigor,
int);
123 # ifndef ITK_USE_CUFFTW
134 UpdateOutputData(
DataObject * output)
override;
137 BeforeThreadedGenerateData()
override;
140 DynamicThreadedGenerateData(
const OutputImageRegionType & outputRegionForThread)
override;
143 PrintSelf(std::ostream & os,
Indent indent)
const override;
154 # ifndef ITK_MANUAL_INSTANTIATION
155 # include "itkFFTWComplexToComplexFFTImageFilter.hxx"
158 #endif // itkFFTWComplexToComplexFFTImageFilter_h
bool m_CanUseDestructiveAlgorithm
virtual void SetPlanRigor(const int &value)
typename ImageType::SizeType ImageSizeType
typename fftw::Proxy< typename PixelType::value_type > FFTWProxyType
ImageBaseType::SizeType SizeType
Control indentation during Print() invocation.
Implements an API to enable the Fourier transform or the inverse Fourier transform of images with com...
static std::string GetPlanRigorName(const int &value)
Light weight base class for most itk classes.
void SetPlanRigor(const std::string &name)
ImageBaseType::RegionType RegionType
static int GetPlanRigorValue(const std::string &name)
Implements an API to enable the Fourier transform or the inverse Fourier transform of images with com...
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename ImageType::PixelType PixelType
typename OutputImageType::RegionType OutputImageRegionType
Base class for all data objects in ITK.