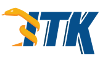 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
19 #ifndef itkFastMarchingReachedTargetNodesStoppingCriterion_h
20 #define itkFastMarchingReachedTargetNodesStoppingCriterion_h
24 #include "ITKFastMarchingExport.h"
47 extern ITKFastMarching_EXPORT std::ostream &
59 template <
typename TInput,
typename TOutput>
66 using Superclass = FastMarchingStoppingCriterionBase<TInput, TOutput>;
69 using Traits =
typename Superclass::Traits;
78 using NodeType =
typename Superclass::NodeType;
81 #if !defined(ITK_LEGACY_REMOVE)
139 typename std::vector<NodeType>::const_iterator pointsIter =
m_TargetNodes.begin();
140 typename std::vector<NodeType>::const_iterator pointsEnd =
m_TargetNodes.end();
142 while (pointsIter != pointsEnd)
144 if (*pointsIter == iNode)
175 return "Target Nodes Reached with possible overshoot";
217 itkExceptionMacro(<<
"Number of target nodes to be reached is null");
222 <<
"Number of target nodes to be reached is above the provided number of target nodes");
231 #endif // itkFastMarchingThresholdStoppingCriterion_h
bool IsSatisfied() const override
returns if the stopping condition is satisfied or not.
OutputPixelType m_TargetOffset
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
~FastMarchingReachedTargetNodesStoppingCriterion() override=default
FastMarchingReachedTargetNodesStoppingCriterion()
std::vector< NodeType > m_ReachedTargetNodes
void SetCurrentNode(const NodeType &iNode) override
Set the current node.
typename Superclass::OutputPixelType OutputPixelType
FastMarchingStoppingCriterionBase< TInput, TOutput > Superclass
std::vector< NodeType > m_TargetNodes
Define additional traits for native types such as int or float.
TargetConditionEnum m_TargetCondition
void SetNumberOfTargetsToBeReached(const vcl_size_t &iN)
Set the number of target nodes to be reached.
std::string GetDescription() const override
Get a short description of the stopping criterion.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Stopping criterion for FastMarchingFilterBase.
Contains all enum classes used by FastMarchingReachedTargetNodesStoppingCriterion class.
FastMarchingReachedTargetNodesStoppingCriterionEnums::TargetCondition TargetConditionEnum
virtual void SetTargetNodes(const std::vector< NodeType > &iNodes)
Set Target Nodes.
void SetTargetCondition(const TargetConditionEnum &iCondition)
typename Superclass::Traits Traits
vcl_size_t m_NumberOfTargetsToBeReached
OutputPixelType m_StoppingValue
typename Superclass::NodeType NodeType