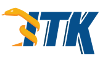 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
19 #ifndef itkImageBufferRange_h
20 #define itkImageBufferRange_h
26 #include <type_traits>
72 template <
typename TImage>
86 std::is_same<PixelType, InternalPixelType>::value &&
87 std::is_same<typename TImage::AccessorType, DefaultPixelAccessor<PixelType>>::value &&
88 std::is_same<AccessorFunctorType, DefaultPixelAccessorFunctor<typename std::remove_const<TImage>::type>>::value;
97 typename std::conditional<SupportsDirectPixelAccess, EmptyAccessorFunctor, AccessorFunctorType>::type;
106 template <
bool VIsConst,
typename TDummy =
void>
112 template <
typename TDummy>
134 : m_InternalPixel{ internalPixel }
135 , m_AccessorFunctor(accessorFunctor)
140 : m_InternalPixel{ pixelProxy.m_InternalPixel }
141 , m_AccessorFunctor{ pixelProxy.m_AccessorFunctor }
145 operator PixelType() const ITK_NOEXCEPT {
return m_AccessorFunctor.Get(m_InternalPixel); }
151 template <
typename TDummy>
175 : m_InternalPixel{ internalPixel }
176 , m_AccessorFunctor(accessorFunctor)
180 operator PixelType() const ITK_NOEXCEPT {
return m_AccessorFunctor.Get(m_InternalPixel); }
186 m_AccessorFunctor.Set(m_InternalPixel, pixelValue);
205 const auto lhsPixelValue = lhs.m_AccessorFunctor.Get(lhs.m_InternalPixel);
206 const auto rhsPixelValue = rhs.m_AccessorFunctor.Get(rhs.m_InternalPixel);
209 lhs.m_AccessorFunctor.Set(lhs.m_InternalPixel, rhsPixelValue);
210 rhs.m_AccessorFunctor.Set(rhs.m_InternalPixel, lhsPixelValue);
227 template <
bool VIsConst>
245 typename std::conditional<IsImageTypeConst, const InternalPixelType, InternalPixelType>::type;
248 using QualifiedPixelType =
typename std::conditional<IsImageTypeConst, const PixelType, PixelType>::type;
296 typename std::conditional<SupportsDirectPixelAccess, QualifiedPixelType &, PixelProxy<IsImageTypeConst>>::type;
330 using PixelWrapper =
typename std::conditional<SupportsDirectPixelAccess, PixelReferenceWrapper, reference>::type;
388 return lhs.m_InternalPixelPointer == rhs.m_InternalPixelPointer;
397 return !(lhs == rhs);
405 return lhs.m_InternalPixelPointer < rhs.m_InternalPixelPointer;
458 return lhs.m_InternalPixelPointer - rhs.m_InternalPixelPointer;
498 typename std::conditional<IsImageTypeConst, const InternalPixelType, InternalPixelType>::type;
513 result.SetPixelAccessor(
m_Image.GetPixelAccessor());
514 result.SetBegin(
m_Image.ImageType::GetBufferPointer());
536 template <
bool VIsConst>
560 typename std::conditional<UsingPointerAsIterator, const InternalPixelType *, QualifiedIterator<true>>::type;
562 conditional<UsingPointerAsIterator, QualifiedInternalPixelType *, QualifiedIterator<IsImageTypeConst>>::type;
582 ,
m_NumberOfPixels{ image.ImageType::GetBufferedRegion().GetNumberOfPixels() }
609 return this->
begin();
670 assert(n < this->
size());
671 assert(n <= static_cast<std::size_t>(std::numeric_limits<std::ptrdiff_t>::max()));
674 return this->
begin()[static_cast<std::ptrdiff_t>(n)];
682 template <
typename TImage>
683 ImageBufferRange<TImage>
686 if (image ==
nullptr)
bool empty() const noexcept
PixelProxy(const InternalPixelType &internalPixel, const AccessorFunctorType &accessorFunctor) noexcept
QualifiedIterator()=default
InternalPixelType & m_InternalPixel
QualifiedInternalPixelType * m_ImageBufferPointer
friend bool operator>(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
IteratorInitializer(OptionalAccessorFunctorType optionalAccessorFunctor, QualifiedInternalPixelType *internalPixelPointer) noexcept
reverse_iterator rend() const noexcept
std::vcl_size_t size() const noexcept
typename TImage::InternalPixelType InternalPixelType
QualifiedIterator(const QualifiedIterator< false > &arg) noexcept
OptionalAccessorFunctorType m_OptionalAccessorFunctor
friend bool operator>=(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
const_reverse_iterator crend() const noexcept
reference operator[](const difference_type n) const noexcept
QualifiedIterator & operator=(const QualifiedIterator &) noexcept=default
friend QualifiedIterator operator+(QualifiedIterator it, const difference_type n) noexcept
SizeValueType m_NumberOfPixels
typename std::conditional< UsingPointerAsIterator, const InternalPixelType *, QualifiedIterator< true > >::type const_iterator
QualifiedInternalPixelType * m_InternalPixelPointer
PixelProxy(const PixelProxy< false > &pixelProxy) noexcept
QualifiedIterator(const OptionalAccessorFunctorType &accessorFunctor, QualifiedInternalPixelType *const internalPixelPointer) noexcept
typename std::conditional< VIsConst, const ImageType, ImageType >::type QualifiedImageType
QualifiedIterator & operator--() noexcept
friend void swap(PixelProxy lhs, PixelProxy rhs) noexcept
friend QualifiedIterator operator-(QualifiedIterator it, const difference_type n) noexcept
typename TImage::PixelType PixelType
typename std::conditional< IsImageTypeConst, const PixelType, PixelType >::type QualifiedPixelType
const AccessorFunctorType m_AccessorFunctor
static constexpr bool IsImageTypeConst
iterator end() const noexcept
PixelProxy & operator=(const PixelProxy &pixelProxy) noexcept
friend QualifiedIterator & operator+=(QualifiedIterator &it, const difference_type n) noexcept
OptionalAccessorFunctorType m_OptionalAccessorFunctor
ImageBufferRange< TImage > MakeImageBufferRange(TImage *const image)
QualifiedIterator operator++(int) noexcept
std::reverse_iterator< const_iterator > const_reverse_iterator
std::ptrdiff_t difference_type
typename std::conditional< IsImageTypeConst, const InternalPixelType, InternalPixelType >::type QualifiedInternalPixelType
friend bool operator<=(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
constexpr static bool UsingPointerAsIterator
iterator begin() const noexcept
std::reverse_iterator< iterator > reverse_iterator
ImageBufferRange()=default
QualifiedInternalPixelType * m_InternalPixelPointer
typename std::conditional< UsingPointerAsIterator, QualifiedInternalPixelType *, QualifiedIterator< IsImageTypeConst > >::type iterator
friend QualifiedIterator & operator-=(QualifiedIterator &it, const difference_type n) noexcept
QualifiedPixelType * pointer
PixelProxy(InternalPixelType &internalPixel, const AccessorFunctorType &accessorFunctor) noexcept
friend bool operator<(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
const AccessorFunctorType m_AccessorFunctor
friend difference_type operator-(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
QualifiedIterator operator--(int) noexcept
typename std::conditional< IsImageTypeConst, const InternalPixelType, InternalPixelType >::type QualifiedInternalPixelType
static constexpr bool IsImageTypeConst
friend bool operator==(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename std::conditional< SupportsDirectPixelAccess, EmptyAccessorFunctor, AccessorFunctorType >::type OptionalAccessorFunctorType
typename std::conditional< SupportsDirectPixelAccess, QualifiedPixelType &, PixelProxy< IsImageTypeConst > >::type reference
const_iterator cend() const noexcept
const InternalPixelType & m_InternalPixel
reverse_iterator rbegin() const noexcept
AccessorFunctorInitializer(ImageType &image) noexcept
QualifiedIterator & operator++() noexcept
constexpr static bool SupportsDirectPixelAccess
reference operator*() const noexcept
std::random_access_iterator_tag iterator_category
PixelReferenceWrapper(QualifiedPixelType &pixel, EmptyAccessorFunctor) noexcept
PixelProxy & operator=(const PixelType &pixelValue) noexcept
typename TImage::AccessorFunctorType AccessorFunctorType
const_reverse_iterator crbegin() const noexcept
const_iterator cbegin() const noexcept
friend bool operator!=(const QualifiedIterator &lhs, const QualifiedIterator &rhs) noexcept
QualifiedPixelType & m_Pixel
ImageBufferRange(ImageType &image)
unsigned long SizeValueType
friend QualifiedIterator operator+(const difference_type n, QualifiedIterator it) noexcept
QualifiedIterator< false >::reference operator[](const std::vcl_size_t n) const noexcept
OptionalAccessorFunctorType m_OptionalAccessorFunctor