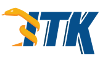 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkImageHelper_h
19 #define itkImageHelper_h
22 #include <type_traits>
50 template <
unsigned int NImageDimension>
53 template <
unsigned int NImageDimension,
unsigned int NLoop>
71 bufferedRegionIndex, offset, offsetTable, index, std::integral_constant<bool, NLoop == 1>{});
82 index[NLoop] = static_cast<IndexValueType>(offset / offsetTable[NLoop]);
83 offset -= (index[NLoop] * offsetTable[NLoop]);
84 index[NLoop] += bufferedRegionIndex[NLoop];
86 bufferedRegionIndex, offset, offsetTable, index, std::integral_constant<bool, NLoop == 1>{});
97 index[0] = bufferedRegionIndex[0] + static_cast<IndexValueType>(offset);
109 bufferedRegionIndex, index, offsetTable, offset, std::integral_constant<bool, NLoop == 1>{});
119 offset += (index[NLoop] - bufferedRegionIndex[NLoop]) * offsetTable[NLoop];
121 bufferedRegionIndex, index, offsetTable, offset, std::integral_constant<bool, NLoop == 1>{});
132 offset += index[0] - bufferedRegionIndex[0];
static void ComputeOffsetInner(const IndexType &bufferedRegionIndex, const IndexType &index, const OffsetValueType offsetTable[], OffsetValueType &offset, std::false_type)
typename OffsetType::OffsetValueType OffsetValueType
static void ComputeOffsetInner(const IndexType &bufferedRegionIndex, const IndexType &index, const OffsetValueType[], OffsetValueType &offset, std::true_type)
Base class for templated image classes.
typename ImageType::OffsetValueType OffsetValueType
typename IndexType::IndexValueType IndexValueType
Index< VImageDimension > IndexType
Offset< VImageDimension > OffsetType
static void ComputeIndexInner(const IndexType &bufferedRegionIndex, OffsetValueType &offset, const OffsetValueType offsetTable[], IndexType &index, std::false_type)
typename ImageType::OffsetType OffsetType
typename ImageType::IndexValueType IndexValueType
typename ImageType::IndexType IndexType
Fast Index/Offset computation.
static void ComputeIndexInner(const IndexType &bufferedRegionIndex, OffsetValueType &offset, const OffsetValueType[], IndexType &index, std::true_type)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
static void ComputeIndex(const IndexType &bufferedRegionIndex, OffsetValueType offset, const OffsetValueType offsetTable[], IndexType &index)
static void ComputeOffset(const IndexType &bufferedRegionIndex, const IndexType &index, const OffsetValueType offsetTable[], OffsetValueType &offset)