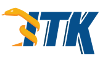 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
19 #ifndef itkLevelSetContainer_h
20 #define itkLevelSetContainer_h
38 template <
typename TIdentifier,
typename TLevelSet>
92 template <
typename TIdentifier,
typename TImage>
152 while (it != internalContainer.end())
161 image->CopyInformation(otherImage);
162 image->SetBufferedRegion(otherImage->GetBufferedRegion());
163 image->SetRequestedRegion(otherImage->GetRequestedRegion());
164 image->SetLargestPossibleRegion(otherImage->GetLargestPossibleRegion());
168 temp_ls->SetImage(image);
169 newContainer[it->first] = temp_ls;
170 newContainer[it->first]->SetDomainOffset((it->second)->GetDomainOffset());
175 newContainer[it->first] = temp_ls;
190 #endif // itkLevelSetContainer_h
typename Superclass::HessianType HessianType
typename HeavisideType::ConstPointer HeavisideConstPointer
typename Superclass::HeavisideConstPointer HeavisideConstPointer
typename Superclass::IdListIterator IdListIterator
typename Superclass::LevelSetContainerIteratorType LevelSetContainerIteratorType
typename Superclass::LevelSetPointer LevelSetPointer
typename Superclass::GradientType GradientType
std::map< LevelSetIdentifierType, LevelSetPointer > LevelSetContainerType
typename LevelSetImageType::Pointer LevelSetImagePointer
HeavisideStepFunctionBase< OutputRealType, OutputRealType > HeavisideType
typename Superclass::DomainIteratorType DomainIteratorType
typename Superclass::LevelSetContainerConstIteratorType LevelSetContainerConstIteratorType
Base class for the "dense" representation of a level-set function on one image.
typename Superclass::IdListImageType IdListImageType
typename Superclass::OutputType OutputPixelType
typename Superclass::LevelSetContainerType LevelSetContainerType
typename DomainMapImageFilterType::LevelSetDomain LevelSetDomainType
void SetContainer(const LevelSetContainerType &iContainer)
typename DomainMapImageFilterType::Pointer DomainMapImageFilterPointer
typename Superclass::OutputType OutputPixelType
std::list< LevelSetIdentifierType > IdListType
typename Superclass::HeavisideType HeavisideType
typename Superclass::CacheImageType CacheImageType
typename Superclass::LevelSetDomainType LevelSetDomainType
typename Superclass::DomainMapImageFilterPointer DomainMapImageFilterPointer
LevelSetContainer()=default
typename Superclass::InputIndexType InputIndexType
typename Superclass::GradientType GradientType
typename Superclass::IdListType IdListType
Light weight base class for most itk classes.
typename Superclass::LevelSetIdentifierType LevelSetIdentifierType
typename LevelSetType::OutputType OutputType
typename Superclass::DomainMapImageFilterType DomainMapImageFilterType
void CopyInformationAndAllocate(const Self *iOther, const bool &iAllocate)
typename LevelSetContainerType::const_iterator LevelSetContainerConstIteratorType
typename Superclass::LevelSetContainerIteratorType LevelSetContainerIteratorType
typename Superclass::HeavisideType HeavisideType
typename LevelSetType::InputType InputIndexType
typename Superclass::HeavisideConstPointer HeavisideConstPointer
typename Superclass::DomainIteratorType DomainIteratorType
Image< short, Dimension > CacheImageType
typename LevelSetType::GradientType GradientType
static constexpr unsigned int Dimension
typename Superclass::IdListIterator IdListIterator
typename Superclass::CacheImageType CacheImageType
typename DomainContainerType::iterator DomainIteratorType
typename Superclass::HessianType HessianType
Define additional traits for native types such as int or float.
TIdentifier LevelSetIdentifierType
typename LevelSetType::HessianType HessianType
typename Superclass::LevelSetDomainType LevelSetDomainType
typename Superclass::OutputRealType OutputRealType
typename LevelSetType::OutputRealType OutputRealType
typename Superclass::LevelSetIdentifierType LevelSetIdentifierType
LevelSetDomainMapImageFilter< IdListImageType, CacheImageType > DomainMapImageFilterType
typename Superclass::IdListType IdListType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename LevelSetContainerType::iterator LevelSetContainerIteratorType
typename Superclass::LevelSetType LevelSetType
typename IdListType::iterator IdListIterator
~LevelSetContainer() override=default
typename Superclass::LevelSetPointer LevelSetPointer
typename LevelSetType::Pointer LevelSetPointer
Image< IdListType, Dimension > IdListImageType
typename Superclass::OutputRealType OutputRealType
typename Superclass::InputIndexType InputIndexType
constexpr unsigned int Dimension
typename Superclass::DomainMapImageFilterPointer DomainMapImageFilterPointer
typename Superclass::LevelSetContainerConstIteratorType LevelSetContainerConstIteratorType
typename LevelSetType::ImageType LevelSetImageType
typename Superclass::IdListImageType IdListImageType
typename Superclass::LevelSetContainerType LevelSetContainerType
typename Superclass::DomainMapImageFilterType DomainMapImageFilterType