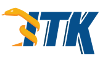 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkLogicOpsFunctors_h
19 #define itkLogicOpsFunctors_h
58 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
81 return !(*
this != other);
87 m_ForegroundValue = FG;
92 m_BackgroundValue = BG;
98 return (m_ForegroundValue);
103 return (m_BackgroundValue);
121 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
138 return !(*
this != other);
145 return this->m_ForegroundValue;
147 return this->m_BackgroundValue;
160 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
176 return !(*
this != other);
183 return this->m_ForegroundValue;
185 return this->m_BackgroundValue;
199 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
215 return !(*
this != other);
222 return this->m_ForegroundValue;
224 return this->m_BackgroundValue;
238 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
253 return !(*
this != other);
260 return this->m_ForegroundValue;
262 return this->m_BackgroundValue;
277 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
293 return !(*
this != other);
300 return this->m_ForegroundValue;
302 return this->m_BackgroundValue;
316 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
331 return !(*
this != other);
338 return this->m_ForegroundValue;
340 return this->m_BackgroundValue;
351 template <
typename TInput,
typename TOutput = TInput>
367 return !(*
this != other);
375 return this->m_ForegroundValue;
377 return this->m_BackgroundValue;
386 template <
typename TInput1,
typename TInput2,
typename TInput3,
typename TOutput>
402 return !(*
this != other);
406 operator()(
const TInput1 & A,
const TInput2 & B,
const TInput3 & C)
const
410 return static_cast<TOutput>(B);
414 return static_cast<TOutput>(C);
Functor for > operation on images and constants.
TOutput operator()(const TInput1 &A, const TInput2 &B) const
Functor for >= operation on images and constants.
bool operator!=(const Self &) const
bool operator==(const Self &other) const
TOutput operator()(const TInput1 &A, const TInput2 &B, const TInput3 &C) const
TOutput operator()(const TInput &A) const
TOutput GetForegroundValue() const
bool operator!=(const TernaryOperator &) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const
Base class for some logic functors. Provides the Foreground and background setting methods.
Unary logical NOT functor.
bool ExactlyEquals(const TInput1 &x1, const TInput2 &x2)
Return the result of an exact comparison between two scalar values of potentially different types.
bool operator!=(const Self &) const
bool operator==(const Self &other) const
bool operator==(const Self &other) const
bool NotExactlyEquals(const TInput1 &x1, const TInput2 &x2)
void SetForegroundValue(const TOutput &FG)
bool operator!=(const Self &) const
bool operator!=(const Self &) const
TOutput m_ForegroundValue
TOutput operator()(const TInput1 &A, const TInput2 &B) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const
TOutput m_BackgroundValue
Functor for <= operation on images and constants.
Functor for == operation on images and constants.
Return argument 2 if argument 1 is false, and argument 3 otherwise.
bool operator!=(const NOT &) const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
TOutput operator()(const TInput1 &A, const TInput2 &B) const
bool operator==(const Self &other) const
bool operator!=(const Self &) const
Functor for != operation on images and constants.
TOutput operator()(const TInput1 &A, const TInput2 &B) const
TOutput GetBackgroundValue() const
bool operator!=(const Self &) const
bool operator==(const Self &other) const
Functor for < operation on images and constants.
bool operator==(const NOT &other) const
bool operator!=(const Self &) const
bool operator==(const TernaryOperator &other) const
void SetBackgroundValue(const TOutput &BG)
bool operator==(const Self &other) const
bool operator==(const Self &other) const