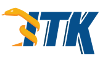 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkMRFImageFilter_h
19 #define itkMRFImageFilter_h
21 #include "vnl/vnl_vector.h"
22 #include "vnl/vnl_matrix.h"
31 #include "ITKMarkovRandomFieldsClassifiersExport.h"
55 extern ITKMarkovRandomFieldsClassifiers_EXPORT std::ostream &
148 template <
typename TInputImage,
typename TClassifiedImage>
184 static constexpr
unsigned int InputImageDimension = TInputImage::ImageDimension;
215 static constexpr
unsigned int ClassifiedImageDimension = TClassifiedImage::ImageDimension;
253 itkSetMacro(NumberOfClasses,
unsigned int);
254 itkGetConstMacro(NumberOfClasses,
unsigned int);
259 itkSetMacro(MaximumNumberOfIterations,
unsigned int);
260 itkGetConstMacro(MaximumNumberOfIterations,
unsigned int);
265 itkSetMacro(ErrorTolerance,
double);
266 itkGetConstMacro(ErrorTolerance,
double);
271 itkSetMacro(SmoothingFactor,
double);
272 itkGetConstMacro(SmoothingFactor,
double);
294 for (
int i = 0; i < InputImageDimension; ++i)
296 radius[i] = m_InputImageNeighborhoodRadius[i];
307 SetMRFNeighborhoodWeight(std::vector<double> betaMatrix);
309 virtual std::vector<double>
312 return m_MRFNeighborhoodWeight;
316 #if !defined(ITK_LEGACY_REMOVE)
320 static constexpr
MRFStopEnum MaximumNumberOfIterations = MRFStopEnum::MaximumNumberOfIterations;
321 static constexpr
MRFStopEnum ErrorTolerance = MRFStopEnum::ErrorTolerance;
329 itkGetConstReferenceMacro(NumberOfIterations,
unsigned int);
331 #ifdef ITK_USE_CONCEPT_CHECKING
347 PrintSelf(std::ostream & os,
Indent indent)
const override;
358 ApplyMRFImageFilter();
362 MinimizeFunctional();
380 GenerateData()
override;
383 GenerateInputRequestedRegion()
override;
386 EnlargeOutputRequestedRegion(
DataObject *)
override;
389 GenerateOutputInformation()
override;
406 unsigned int m_NumberOfClasses{ 0 };
407 unsigned int m_MaximumNumberOfIterations{ 50 };
410 int m_ErrorCounter{ 0 };
411 int m_NeighborhoodSize{ 27 };
412 int m_TotalNumberOfValidPixelsInOutputImage{ 1 };
413 int m_TotalNumberOfPixelsInInputImage{ 1 };
414 double m_ErrorTolerance{ 0.2 };
415 double m_SmoothingFactor{ 1 };
416 double * m_ClassProbability{
nullptr };
417 unsigned int m_NumberOfIterations{ 0 };
418 MRFStopEnum m_StopCondition{ MRFStopEnum::MaximumNumberOfIterations };
434 SetDefaultMRFNeighborhoodWeight();
442 #ifndef ITK_MANUAL_INSTANTIATION
443 # include "itkMRFImageFilter.hxx"
Splits an image into a main region and several "face" regions which are used to handle computations o...
std::vector< double > m_MahalanobisDistance
LabelledImageNeighborhoodRadiusType m_LabelledImageNeighborhoodRadius
std::vector< double > m_DummyVector
Implementation of a labeller object that uses Markov Random Fields to classify pixels in an image dat...
typename LabelledImageNeighborhoodIterator::RadiusType LabelledImageNeighborhoodRadiusType
typename LabelStatusImageType::IndexType LabelStatusIndexType
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
typename TInputImage::ConstPointer InputImageConstPointer
typename InputImageNeighborhoodIterator::RadiusType InputImageNeighborhoodRadiusType
typename LabelledImageFacesCalculator::FaceListType LabelledImageFaceListType
ImageBaseType::SizeType SizeType
Contains all enum classes in MRFImageFilter class;.
typename LabelStatusImageFaceListType::iterator LabelStatusImageFaceListIterator
Control indentation during Print() invocation.
typename LabelStatusImageNeighborhoodIterator::RadiusType LabelStatusImageNeighborhoodRadiusType
typename TClassifiedImage::Pointer LabelledImagePointer
std::vector< double > m_MRFNeighborhoodWeight
LabelStatusImagePointer m_LabelStatusImage
std::vector< double > m_NeighborInfluence
typename TClassifiedImage::Pointer TrainingImagePointer
A multi-dimensional iterator templated over image type that walks a region of pixels.
ImageBaseType::IndexType IndexType
typename LabelStatusImageFacesCalculator::FaceListType LabelStatusImageFaceListType
Base class for filters that take an image as input and produce an image as output.
typename Superclass::OutputImagePointer OutputImagePointer
Light weight base class for most itk classes.
typename LabelledImageIndexType::IndexValueType IndexValueType
typename TClassifiedImage::IndexType LabelledImageIndexType
typename TInputImage::RegionType InputImageRegionType
LabelStatusImageNeighborhoodRadiusType m_LabelStatusImageNeighborhoodRadius
Base class for the ImageClassifierBase object.
ImageBaseType::RegionType RegionType
typename TInputImage::SizeType InputImageSizeType
typename Superclass::RadiusType RadiusType
typename LabelStatusImageType::Pointer LabelStatusImagePointer
typename TInputImage::PixelType InputImagePixelType
virtual std::vector< double > GetMRFNeighborhoodWeight()
typename TInputImage::Pointer InputImagePointer
const NeighborhoodRadiusType GetNeighborhoodRadius() const
typename InputImageFaceListType::iterator InputImageFaceListIterator
typename TClassifiedImage::RegionType LabelledImageRegionType
ClassifierType::Pointer m_ClassifierPtr
typename InputImageFacesCalculator::FaceListType InputImageFaceListType
TInputImage InputImageType
Defines iteration of a local N-dimensional neighborhood of pixels across an itk::Image.
typename TInputImage::SizeType NeighborhoodRadiusType
#define itkConceptMacro(name, concept)
std::list< RegionType > FaceListType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename TInputImage::SizeType SizeType
InputImageNeighborhoodRadiusType m_InputImageNeighborhoodRadius
signed long IndexValueType
Base class for most ITK classes.
typename TClassifiedImage::PixelType LabelledImagePixelType
A multi-dimensional iterator templated over image type that walks a region of pixels.
Templated n-dimensional image class.
typename TClassifiedImage::OffsetType LabelledImageOffsetType
typename TClassifiedImage::PixelType TrainingImagePixelType
unsigned int m_KernelSize
typename LabelStatusImageType::RegionType LabelStatusRegionType
typename LabelledImageFaceListType::iterator LabelledImageFaceListIterator
unsigned long SizeValueType
Base class for all data objects in ITK.