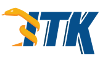 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkMaskNegatedImageFilter_h
19 #define itkMaskNegatedImageFilter_h
34 template <
typename TInput,
typename TMask,
typename TOutput = TInput>
54 return !(*
this != other);
66 return static_cast<TOutput>(A);
138 template <
typename TInputImage,
typename TMaskImage,
typename TOutputImage = TInputImage>
149 MaskNegatedInput<typename TInputImage::PixelType, typename TMaskImage::PixelType, typename TOutputImage::PixelType>;
174 const typename TOutputImage::PixelType &
193 const typename TMaskImage::PixelType &
207 this->
SetNthInput(1, const_cast<MaskImageType *>(maskImage));
216 #ifdef ITK_USE_CONCEPT_CHECKING
232 os << indent <<
"OutsideValue: " << this->
GetOutsideValue() << std::endl;
~MaskNegatedImageFilter() override=default
void PrintSelf(std::ostream &os, Indent indent) const override
virtual const FunctorType & GetFunctor() const
Implements pixel-wise generic operation of two images, or of an image and a constant.
Control indentation during Print() invocation.
bool NotExactlyEquals(const TInput1 &x1, const TInput2 &x2)
const TMaskImage::PixelType & GetMaskingValue() const
const TOutputImage::PixelType & GetOutsideValue() const
const MaskImageType * GetMaskImage()
Base class for all process objects that output image data.
void PrintSelf(std::ostream &os, Indent indent) const override
Functor::MaskNegatedInput< typename TInputImage::PixelType, typename TMaskImage::PixelType, typename TOutputImage::PixelType > FunctorType
Mask an image with the negation (or logical compliment) of a mask.
void BeforeThreadedGenerateData() override
void SetOutsideValue(const typename TOutputImage::PixelType &outsideValue)
MaskNegatedImageFilter()=default
Define additional traits for native types such as int or float.
#define itkConceptMacro(name, concept)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
virtual void SetNthInput(DataObjectPointerArraySizeType idx, DataObject *input)
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
FunctorType & GetFunctor()
virtual void Modified() const
DataObject * GetInput(const DataObjectIdentifierType &key)
Return an input.
void SetFunctor(const std::function< ConstRefFunctionType > &f)
void SetMaskImage(const MaskImageType *maskImage)
void SetMaskingValue(const typename TMaskImage::PixelType &maskingValue)