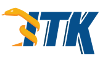 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkNthElementPixelAccessor_h
19 #define itkNthElementPixelAccessor_h
52 template <
typename T,
typename TContainer>
119 template <
typename TOutputPixelType,
typename TPixelType>
151 return Set(v, input);
166 return Get(Superclass::Get(input, offset));
188 Superclass::SetVectorLength(l);
195 return Superclass::GetVectorLength();
213 this->SetVectorLength(accessor.GetVectorLength());
ExternalType Get(const ActualPixelType &input) const
NthElementPixelAccessor & operator=(const NthElementPixelAccessor &accessor)
void SetVectorLength(VectorLengthType l)
unsigned int VectorLengthType
void Set(InternalType &output, const ExternalType &input, const unsigned long offset) const
bool operator!=(const Self &accessor) const
void SetElementNumber(unsigned int nth)
ExternalType Get(const InternalType &input, const SizeValueType offset) const
void Set(ActualPixelType &output, const ExternalType &input) const
NthElementPixelAccessor()
Give access to partial aspects of a type.
Traits class used to by ConvertPixels to convert blocks of pixels.
static void SetNthComponent(int c, PixelType &pixel, const ComponentType &v)
ExternalType Get(const InternalType &input) const
NthElementPixelAccessor & operator=(const NthElementPixelAccessor &accessor)
void Set(InternalType &output, const ExternalType &input) const
void SetElementNumber(unsigned int nth)
Give access to the N-th of a Container type.
Represents an array whose length can be defined at run-time.
unsigned int GetElementNumber() const
unsigned int m_ElementNumber
TOutputPixelType ExternalType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
VectorLengthType GetVectorLength() const
unsigned int GetElementNumber() const
bool operator!=(const Self &accessor) const
NthElementPixelAccessor(unsigned int length=1)
unsigned long SizeValueType