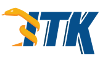 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkVideoStream_h
19 #define itkVideoStream_h
41 template <
typename TFrameType>
74 static constexpr
unsigned int FrameDimension = FrameType::ImageDimension;
78 return FrameType::ImageDimension;
97 InitializeEmptyFrames();
103 return reinterpret_cast<BufferType *>(m_DataObjectBuffer.GetPointer());
108 return reinterpret_cast<BufferType *>(m_DataObjectBuffer.GetPointer());
114 SetFrameBuffer(BufferType * buffer);
117 const SpatialRegionMapType &
120 return m_LargestPossibleSpatialRegionCache;
125 m_LargestPossibleSpatialRegionCache = map;
129 const SpatialRegionMapType &
132 return m_RequestedSpatialRegionCache;
137 m_RequestedSpatialRegionCache = map;
140 const SpatialRegionMapType &
143 return m_BufferedSpatialRegionCache;
148 m_BufferedSpatialRegionCache = map;
151 const SpacingMapType &
154 return m_SpacingCache;
159 m_SpacingCache = map;
165 return m_OriginCache;
173 const DirectionMapType &
176 return m_DirectionCache;
181 m_DirectionCache = map;
200 SetFrameLargestPossibleSpatialRegion(
SizeValueType frameNumber, SpatialRegionType region);
202 const SpatialRegionType &
203 GetFrameLargestPossibleSpatialRegion(
SizeValueType frameNumber)
const;
207 SetFrameRequestedSpatialRegion(
SizeValueType frameNumber, SpatialRegionType region);
209 const SpatialRegionType &
210 GetFrameRequestedSpatialRegion(
SizeValueType frameNumber)
const;
214 SetFrameBufferedSpatialRegion(
SizeValueType frameNumber, SpatialRegionType region);
216 const SpatialRegionType &
217 GetFrameBufferedSpatialRegion(
SizeValueType frameNumber)
const;
221 SetFrameSpacing(
SizeValueType frameNumber, SpacingType spacing);
244 SetAllLargestPossibleSpatialRegions(SpatialRegionType region);
250 SetAllRequestedSpatialRegions(SpatialRegionType region);
256 SetAllBufferedSpatialRegions(SpatialRegionType region);
262 SetAllFramesSpacing(SpacingType spacing);
326 Superclass::Print(os, indent);
345 #ifndef ITK_MANUAL_INSTANTIATION
346 # include "itkVideoStream.hxx"
class ITK_FORWARD_EXPORT TemporalDataObject
typename FrameType::PointType PointType
SpatialRegionMapType m_RequestedSpatialRegionCache
SpatialRegionMapType m_BufferedSpatialRegionCache
typename std::map< SizeValueType, SpacingType > SpacingMapType
ImageBaseType::DirectionType DirectionType
BufferType * GetFrameBuffer()
ImageBaseType::PointType PointType
typename FrameType::RegionType SpatialRegionType
const SpatialRegionMapType & GetRequestedSpatialRegionCache() const
typename FrameType::PixelType PixelType
const SpacingMapType & GetSpacingCache() const
ImageBaseType::SizeType SizeType
DirectionMapType m_DirectionCache
typename std::map< SizeValueType, SpatialRegionType > SpatialRegionMapType
PointMapType m_OriginCache
Control indentation during Print() invocation.
const SpatialRegionMapType & GetLargestPossibleSpatialRegionCache() const
SpatialRegionMapType m_LargestPossibleSpatialRegionCache
typename FrameType::IndexType IndexType
typename FrameType::SizeType SizeType
void PrintSelf(std::ostream &os, Indent indent) const override
static unsigned int GetFrameDimension()
ImageBaseType::IndexType IndexType
typename std::map< SizeValueType, DirectionType > DirectionMapType
typename std::map< SizeValueType, PointType > PointMapType
ImageBaseType::RegionType RegionType
const SpatialRegionMapType & GetBufferedSpatialRegionCache() const
Templated ring buffer for holding anything.
const PointMapType & GetOriginCache() const
void SetLargestPossibleSpatialRegionCache(SpatialRegionMapType map)
SpacingMapType m_SpacingCache
const DirectionMapType & GetDirectionCache() const
const BufferType * GetFrameBuffer() const
void SetDirectionCache(DirectionMapType map)
Implements a weak reference to an object.
typename FrameType::Pointer FramePointer
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
A DataObject that holds a buffered portion of a video.
Base class for most ITK classes.
typename FrameType::SpacingType SpacingType
void SetOriginCache(PointMapType map)
void SetSpacingCache(SpacingMapType map)
typename FrameType::ConstPointer FrameConstPointer
void SetRequestedSpatialRegionCache(SpatialRegionMapType map)
typename FrameType::DirectionType DirectionType
void SetBufferedSpatialRegionCache(SpatialRegionMapType map)
unsigned long SizeValueType
DataObject subclass with knowledge of temporal region.
Base class for all data objects in ITK.