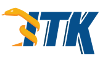 |
ITK
5.2.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkWindowedSincInterpolateImageFunction_h
19 #define itkWindowedSincInterpolateImageFunction_h
36 template <
unsigned int VRadius,
typename TInput =
double,
typename TOutput =
double>
43 return static_cast<TOutput>(std::cos(A * m_Factor));
59 template <
unsigned int VRadius,
typename TInput =
double,
typename TOutput =
double>
66 return static_cast<TOutput>(0.54 + 0.46 * std::cos(A * m_Factor));
82 template <
unsigned int VRadius,
typename TInput =
double,
typename TOutput =
double>
89 return static_cast<TOutput>(1.0 - A * m_Factor * A);
107 template <
unsigned int VRadius,
typename TInput =
double,
typename TOutput =
double>
116 return static_cast<TOutput>(1.0);
118 double z = m_Factor * A;
119 return static_cast<TOutput>(std::sin(z) / z);
135 template <
unsigned int VRadius,
typename TInput =
double,
typename TOutput =
double>
142 return static_cast<TOutput>(0.42 + 0.5 * std::cos(A * m_Factor1) + 0.08 * std::cos(A * m_Factor2));
265 template <
typename TInputImage,
266 unsigned int VRadius,
269 class TCoordRep =
double>
330 radius.Fill(VRadius);
366 return (x == 0.0) ? 1.0 : std::sin(px) / px;
371 #ifndef ITK_MANUAL_INSTANTIATION
372 # include "itkWindowedSincInterpolateImageFunction.hxx"
375 #endif // _itkWindowedSincInterpolateImageFunction_h
TWindowFunction m_WindowFunction
TOutput operator()(const TInput &A) const
typename InputImageType::SizeType SizeType
OutputType EvaluateAtContinuousIndex(const ContinuousIndexType &index) const override
static constexpr unsigned int ImageDimension
void SetInputImage(const ImageType *image) override
TOutput operator()(const TInput &A) const
TOutput operator()(const TInput &A) const
unsigned int m_OffsetTableSize
static const double m_Factor2
~WindowedSincInterpolateImageFunction() override
Window function for sinc interpolation.
Control indentation during Print() invocation.
unsigned int ** m_WeightOffsetTable
static const double m_Factor
Light weight base class for most itk classes.
void PrintSelf(std::ostream &os, Indent indent) const override
static const double m_Factor
unsigned int * m_OffsetTable
typename Superclass::IndexValueType IndexValueType
typename Superclass::ContinuousIndexType ContinuousIndexType
TOutput operator()(const TInput &A) const
double Sinc(double x) const
static const double m_Factor
typename Superclass::InputImageType InputImageType
Window function for sinc interpolation.
Use the windowed sinc function to interpolate.
static constexpr unsigned int ImageDimension
TOutput operator()(const TInput &A) const
typename Superclass::OutputType OutputType
WindowedSincInterpolateImageFunction()
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Const version of NeighborhoodIterator, defining iteration of a local N-dimensional neighborhood of pi...
SizeType GetRadius() const override
static const unsigned int m_WindowSize
A function object that determines a neighborhood of values at an image boundary according to a Neuman...
static constexpr double pi
static const double m_Factor1
Window function for sinc interpolation.
static const double m_Factor
Base class for all image interpolators.
typename Superclass::IndexType IndexType
Window function for sinc interpolation.
Window function for sinc interpolation.
typename NumericTraits< typename TInputImage::PixelType >::RealType RealType