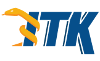 |
ITK
5.3.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkArithmeticOpsFunctors_h
19 #define itkArithmeticOpsFunctors_h
33 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
34 class ITK_TEMPLATE_EXPORT
Add2
46 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Add2);
51 return static_cast<TOutput>(A + B);
61 template <
typename TInput1,
typename TInput2,
typename TInput3,
typename TOutput>
62 class ITK_TEMPLATE_EXPORT
Add3
74 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Add3);
77 operator()(
const TInput1 & A,
const TInput2 & B,
const TInput3 & C)
const
79 return static_cast<TOutput>(A + B + C);
89 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
90 class ITK_TEMPLATE_EXPORT
Sub2
102 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Sub2);
107 return static_cast<TOutput>(A - B);
117 template <
typename TInput1,
typename TInput2 = TInput1,
typename TOutput = TInput1>
130 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Mult);
135 return static_cast<TOutput>(A * B);
145 template <
typename TInput1,
typename TInput2,
typename TOutput>
146 class ITK_TEMPLATE_EXPORT
Div
158 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Div);
165 return (TOutput)(A / B);
180 template <
typename TNumerator,
typename TDenominator = TNumerator,
typename TOutput = TNumerator>
204 operator()(
const TNumerator & n,
const TDenominator & d)
const
210 return static_cast<TOutput>(n) / static_cast<TOutput>(d);
222 template <
typename TInput1,
typename TInput2,
typename TOutput>
236 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(
Modulus);
243 return static_cast<TOutput>(A % B);
252 #if !defined(ITK_FUTURE_LEGACY_REMOVE)
262 template <
typename TInput,
typename TOutput>
263 class ITK_TEMPLATE_EXPORT ModulusTransform
266 ModulusTransform() { m_Dividend = 5; }
267 ~ModulusTransform() =
default;
269 SetDividend(TOutput dividend)
271 m_Dividend = dividend;
276 operator==(
const ModulusTransform & other)
const
278 return m_Dividend == other.m_Dividend;
281 ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(ModulusTransform);
284 operator()(
const TInput & x)
const
286 auto result = static_cast<TOutput>(x % m_Dividend);
306 template <
class TInput1,
class TInput2,
class TOutput>
321 const double temp = std::floor(static_cast<double>(A) / static_cast<double>(B));
322 if (std::is_integral<TOutput>::value && Math::isinf(temp))
333 return static_cast<TOutput>(temp);
348 template <
class TInput1,
class TInput2,
class TOutput>
375 template <
class TInput1,
class TOutput = TInput1>
393 return (TOutput)(-A);
TOutput operator()(const TInput1 &A, const TInput2 &B) const
TOutput operator()(const TInput1 &A) const
bool operator==(const UnaryMinus &) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const
static constexpr T NonpositiveMin()
bool operator==(const Div &) const
ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(DivFloor)
bool operator==(const Add2 &) const
bool operator==(const DivideOrZeroOut &) const
bool operator==(const Add3 &) const
TOutput operator()(const TNumerator &n, const TDenominator &d) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const
TOutput operator()(const TInput1 &A, const TInput2 &B, const TInput3 &C) const
bool NotAlmostEquals(T1 x1, T2 x2)
ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(UnaryMinus)
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
TOutput operator()(const TInput1 &A, const TInput2 &B) const
Cast arguments to double, performs division then takes the floor.
TOutput operator()(const TInput1 &A, const TInput2 &B) const
bool operator==(const Mult &) const
Define additional traits for native types such as int or float.
static constexpr T max(const T &)
bool operator==(const Modulus &) const
Promotes arguments to real type and performs division.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Apply the unary minus operator.
ITK_UNEQUAL_OPERATOR_MEMBER_FUNCTION(DivReal)
static constexpr double e
TOutput operator()(const TInput1 &A, const TInput2 &B) const
bool operator==(const DivFloor &) const
bool operator==(const Sub2 &) const
bool operator==(const DivReal &) const
TOutput operator()(const TInput1 &A, const TInput2 &B) const