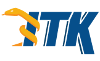 |
ITK
5.3.0
Insight Toolkit
|
Go to the documentation of this file.
26 #include <type_traits>
70 template <
unsigned int VDimension = 2>
71 struct ITK_TEMPLATE_EXPORT
Size final
85 static constexpr
unsigned int Dimension = VDimension;
88 static constexpr
unsigned int
100 for (
unsigned int i = 0; i < VDimension; ++i)
111 for (
unsigned int i = 0; i < VDimension; ++i)
125 for (
unsigned int i = 0; i < VDimension; ++i)
136 for (
unsigned int i = 0; i < VDimension; ++i)
149 for (
unsigned int i = 0; i < VDimension; ++i)
160 for (
unsigned int i = 0; i < VDimension; ++i)
173 return m_InternalArray;
183 std::copy_n(val, VDimension, m_InternalArray);
195 m_InternalArray[element] = val;
207 return m_InternalArray[element];
215 std::fill_n(begin(), size(), value);
232 static_assert(VDimension > 0,
"Error: Only positive value sized VDimension allowed");
259 std::fill_n(begin(), size(), newValue);
268 constexpr const_iterator
271 return &m_InternalArray[0];
277 return &m_InternalArray[0];
280 constexpr const_iterator
283 return &m_InternalArray[0];
286 constexpr const_iterator
289 return &m_InternalArray[VDimension];
295 return &m_InternalArray[VDimension];
298 constexpr const_iterator
301 return &m_InternalArray[VDimension];
310 const_reverse_iterator
322 const_reverse_iterator
353 ExceptionThrowingBoundsCheck(pos);
354 return m_InternalArray[pos];
360 ExceptionThrowingBoundsCheck(pos);
361 return m_InternalArray[pos];
379 return VDimension ? *(end() - 1) : *end();
385 return VDimension ? *(end() - 1) : *end();
391 return &m_InternalArray[0];
397 return &m_InternalArray[0];
404 if (pos >= VDimension)
406 throw std::out_of_range(
"array::ExceptionThrowingBoundsCheck");
413 static constexpr
Self
416 return MakeFilled<Self>(value);
422 template <
unsigned int VDimension>
427 for (
unsigned int i = 0; i + 1 < VDimension; ++i)
429 os << obj[i] <<
", ";
433 os << obj[VDimension - 1];
441 template <
unsigned int VDimension>
448 template <
unsigned int VDimension>
452 return !(one == two);
455 template <
unsigned int VDimension>
459 return std::lexicographical_compare(one.
begin(), one.
end(), two.
begin(), two.
end());
462 template <
unsigned int VDimension>
469 template <
unsigned int VDimension>
476 template <
unsigned int VDimension>
484 template <
unsigned int VDimension>
493 template <
typename... T>
497 const auto toValueType = [](
const auto value) {
498 static_assert(std::is_integral<decltype(value)>::value,
"Each value must have an integral type!");
499 return static_cast<SizeValueType>(value);
501 return Size<
sizeof...(T)>{ { toValueType(values)... } };
507 template <
unsigned int VDimension>
void SetElement(unsigned long element, SizeValueType val)
reverse_iterator rbegin()
bool operator<(const Index< VDimension > &one, const Index< VDimension > &two)
ptrdiff_t difference_type
bool operator<=(const Index< VDimension > &one, const Index< VDimension > &two)
Represent a n-dimensional size (bounds) of a n-dimensional image.
std::ostream & operator<<(std::ostream &os, const Array< TValue > &arr)
void ExceptionThrowingBoundsCheck(size_type pos) const
auto MakeSize(const T... values)
void swap(Array< T > &a, Array< T > &b)
itk::SizeValueType SizeValueType
std::reverse_iterator< iterator > reverse_iterator
static constexpr Self Filled(const SizeValueType value)
constexpr iterator begin()
const Self & operator+=(const Self &vec)
const_reference back() const
const value_type & const_reference
void Fill(SizeValueType value)
constexpr const_iterator begin() const
const_reverse_iterator rbegin() const
const_reverse_iterator rend() const
static constexpr unsigned int GetSizeDimension()
const value_type * const_iterator
constexpr reference operator[](size_type pos)
constexpr const_iterator cend() const
std::reverse_iterator< const_iterator > const_reverse_iterator
const Self & operator*=(const Self &vec)
void assign(const value_type &newValue)
const Self & operator-=(const Self &vec)
constexpr const_iterator cbegin() const
bool operator>=(const Index< VDimension > &one, const Index< VDimension > &two)
bool operator>(const Index< VDimension > &one, const Index< VDimension > &two)
bool operator==(const Index< VDimension > &one, const Index< VDimension > &two)
const Self operator-(const Self &vec) const
constexpr const_reference operator[](size_type pos) const
void SetSize(const SizeValueType val[VDimension])
SizeValueType GetElement(unsigned long element) const
const Self operator*(const Self &vec) const
bool operator!=(const Index< VDimension > &one, const Index< VDimension > &two)
constexpr size_type size() const
SizeValueType m_InternalArray[VDimension]
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
void swap(Size< VDimension > &one, Size< VDimension > &two)
const SizeValueType * data() const
constexpr bool empty() const
const Self operator+(const Self &vec) const
itk::SizeValueType value_type
const_reference front() const
const_reference at(size_type pos) const
constexpr const_iterator end() const
constexpr unsigned int Dimension
constexpr size_type max_size() const
reference at(size_type pos)
static constexpr unsigned int Dimension
unsigned long SizeValueType
const SizeValueType * GetSize() const