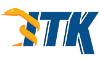 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkAreaClosingImageFilter_h
19 #define itkAreaClosingImageFilter_h
45 template <
typename TInputImage,
46 typename TOutputImage,
47 typename TAttribute =
typename TInputImage::SpacingType::ValueType>
52 std::less<typename TInputImage::PixelType>>
62 std::less<typename TInputImage::PixelType>>;
91 itkSetMacro(UseImageSpacing,
bool);
92 itkGetConstReferenceMacro(UseImageSpacing,
bool);
93 itkBooleanMacro(UseImageSpacing);
107 const auto & spacing = this->
GetInput()->GetSpacing();
127 itkPrintSelfBooleanMacro(UseImageSpacing);
typename TInputImage::OffsetType OffsetType
AttributeType m_AttributeValuePerPixel
typename TInputImage::InternalPixelType InputInternalPixelType
typename TInputImage::IndexType IndexType
typename TInputImage::SizeType SizeType
void GenerateData() override
typename TOutputImage::PixelType OutputPixelType
ImageBaseType::SizeType SizeType
Control indentation during Print() invocation.
typename TOutputImage::InternalPixelType OutputInternalPixelType
typename TInputImage::PixelType InputPixelType
ImageBaseType::IndexType IndexType
Base class for all process objects that output image data.
Morphological opening by attributes.
const InputImageType * GetInput() const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
void GenerateData() override
Morphological closing by attributes.
static constexpr unsigned int ImageDimension
void PrintSelf(std::ostream &os, Indent indent) const override
~AreaClosingImageFilter() override=default
void PrintSelf(std::ostream &os, Indent indent) const override