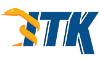 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkAutomaticTopologyMeshSource_h
19 #define itkAutomaticTopologyMeshSource_h
23 #include <unordered_map>
110 template <
typename TOutputMesh>
148 using PointHashMap = std::unordered_map<PointType, IdentifierType, StructHashFunction<PointHashType>>;
151 static constexpr
unsigned int PointDimension = MeshType::PointDimension;
152 static constexpr
unsigned int MaxTopologicalDimension = MeshType::MaxTopologicalDimension;
362 IdType size = identifierArray.
Size();
364 std::sort(identifierArray.begin(), identifierArray.end());
367 IdType *
id = &identifierArray[0];
372 hash = (hash << 7) | (hash >> 25);
387 const IdType size1 = identifierArray1.
Size();
388 const IdType size2 = identifierArray2.
Size();
395 std::sort(identifierArray1.begin(), identifierArray1.end());
396 std::sort(identifierArray2.begin(), identifierArray2.end());
398 return (identifierArray1 == identifierArray2);
412 using CellHashMap = std::
421 #ifndef ITK_MANUAL_INSTANTIATION
422 # include "itkAutomaticTopologyMeshSource.hxx"
425 #endif // itkAutomaticTopologyMeshSource_h
SmartPointer< Self > Pointer
Represents a single vertex for a Mesh.
Represents a quadrilateral for a Mesh.
itk::IdentifierType IdentifierType
typename MeshType::Pointer MeshPointer
ImageBaseType::PointType PointType
Convenience class for generating meshes.
typename CellType::CellAutoPointer CellAutoPointer
std::unordered_map< Array< IdentifierType >, IdentifierType, IdentifierArrayHashFunction, IdentifierArrayEqualsFunction > CellHashMap
TetrahedronCell represents a tetrahedron for a Mesh.
Light weight base class for most itk classes.
Base class for all process objects that output mesh data.
IdentifierType operator()(Array< IdentifierType > identifierArray) const
Represents a hexahedron (cuboid) for a Mesh.
typename MeshType::PointType PointType
Represents a line segment for a Mesh.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename PointType::CoordinateType CoordinateType
bool operator()(Array< IdentifierType > identifierArray1, Array< IdentifierType > identifierArray2) const
SizeValueType Size() const
std::unordered_map< PointType, IdentifierType, StructHashFunction< PointHashType > > PointHashMap
typename MeshType::PointHashType PointHashType
void GenerateData() override
SizeValueType IdentifierType
typename MeshType::CellType CellType