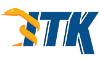 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkBSplineTransformInitializer_h
19 #define itkBSplineTransformInitializer_h
40 template <
typename TTransform,
typename TImage>
80 static constexpr
unsigned int SpaceDimension = TransformType::SpaceDimension;
102 InitializeTransform()
const;
109 PrintSelf(std::ostream & os,
Indent indent)
const override;
116 bool m_SetTransformDomainMeshSizeViaInitializer{
false };
121 #ifndef ITK_MANUAL_INSTANTIATION
122 # include "itkBSplineTransformInitializer.hxx"
SmartPointer< Self > Pointer
SmartPointer< const Self > ConstPointer
ImageBaseType::DirectionType DirectionType
ImageBaseType::PointType PointType
ImageBaseType::SizeType SizeType
Control indentation during Print() invocation.
ImageBaseType::IndexType IndexType
Light weight base class for most itk classes.
ImageBaseType::RegionType RegionType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Base class for most ITK classes.
Templated n-dimensional image class.