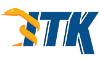 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkCellInterface_h
19 #define itkCellInterface_h
32 #define itkCellVisitMacro(TopologyId) \
33 static constexpr CellGeometryEnum GetTopologyId() { return TopologyId; } \
34 virtual void Accept(CellIdentifier cellid, typename CellInterface<PixelType, CellTraits>::MultiVisitor * mv) \
37 typename CellInterfaceVisitor<PixelType, CellTraits>::Pointer v = mv->GetVisitor(TopologyId); \
40 v->VisitFromCell(cellid, this); \
43 ITK_MACROEND_NOOP_STATEMENT
47 #define itkCellCommonTypedefs(celltype) \
48 using Self = celltype; \
49 using ConstSelfAutoPointer = AutoPointer<const Self>; \
50 using SelfAutoPointer = AutoPointer<Self>; \
51 using RawPointer = Self *; \
52 using ConstRawPointer = const Self *
56 #define itkCellInheritedTypedefs(superclassArg) \
57 using Superclass = superclassArg; \
58 using typename Superclass::PixelType; \
59 using CellType = typename Superclass::CellType; \
60 using typename Superclass::CellAutoPointer; \
61 using typename Superclass::CellConstAutoPointer; \
62 using typename Superclass::CellRawPointer; \
63 using typename Superclass::CellConstRawPointer; \
64 using typename Superclass::CellTraits; \
65 using typename Superclass::CoordinateType; \
66 using typename Superclass::InterpolationWeightType; \
67 using typename Superclass::PointIdentifier; \
68 using typename Superclass::PointIdIterator; \
69 using typename Superclass::PointIdConstIterator; \
70 using typename Superclass::CellIdentifier; \
71 using typename Superclass::CellFeatureIdentifier; \
72 using CellFeatureCount = typename Superclass::CellFeatureIdentifier; \
73 using typename Superclass::PointType; \
74 using typename Superclass::VectorType; \
75 using typename Superclass::PointsContainer; \
76 using typename Superclass::UsingCellsContainer; \
77 using typename Superclass::ParametricCoordArrayType; \
78 using typename Superclass::ShapeFunctionsArrayType; \
79 static constexpr unsigned int PointDimension = Superclass::PointDimension
96 template <
typename TPixelType,
typename TCellTraits>
113 #ifndef ITK_FUTURE_LEGACY_REMOVE
114 using CoordRepType ITK_FUTURE_DEPRECATED(
115 "ITK 6 discourages using `CoordRepType`. Please use `CoordinateType` instead!") =
CoordinateType;
131 static constexpr
unsigned int PointDimension = CellTraits::PointDimension;
195 return m_Visitors[static_cast<int>(
id)];
199 auto pos = m_UserDefined.find(
id);
200 if (pos != m_UserDefined.end())
215 m_Visitors[static_cast<int>(
id)] = v;
249 GetDimension()
const = 0;
253 GetInterpolationOrder()
const;
257 GetNumberOfPoints()
const = 0;
261 GetNumberOfBoundaryFeatures(
int dimension)
const = 0;
298 PointIdsBegin()
const = 0;
307 PointIdsEnd()
const = 0;
312 GetPointIdsContainer()
const;
433 IsExplicitBoundary();
440 AddUsingCell(CellIdentifier cellId);
446 RemoveUsingCell(CellIdentifier cellId);
454 IsUsingCell(CellIdentifier cellId);
460 GetNumberOfUsingCells();
462 #if !defined(ITK_WRAPPING_PARSER)
466 virtual UsingCellsContainerIterator
472 virtual UsingCellsContainerIterator
488 #if !defined(ITK_LEGACY_REMOVE)
527 template <
int VPointDimension,
528 typename TCoordinate,
529 typename TInterpolationWeight,
530 typename TPointIdentifier,
531 typename TCellIdentifier,
532 typename TCellFeatureIdentifier,
534 typename TPointsContainer,
535 typename TUsingCellsContainer>
539 static constexpr
unsigned int PointDimension = VPointDimension;
541 #ifndef ITK_FUTURE_LEGACY_REMOVE
542 using CoordRepType ITK_FUTURE_DEPRECATED(
543 "ITK 6 discourages using `CoordRepType`. Please use `CoordinateType` instead!") =
CoordinateType;
557 #define itkMakeCellTraitsMacro \
558 CellTraitsInfo<Self::PointDimension, \
560 InterpolationWeightType, \
563 CellFeatureIdentifier, \
569 #if !defined(ITK_WRAPPING_PARSER)
570 # ifndef ITK_MANUAL_INSTANTIATION
571 # include "itkCellInterface.hxx"
SmartPointer< Self > Pointer
typename std::map< CellGeometryEnum, VisitorPointer >::value_type VisitorPointerValueType
std::map< CellGeometryEnum, VisitorPointer > m_UserDefined
typename PointType::VectorType VectorType
NOTE: it should normally be defined in the traits.
TPointsContainer PointsContainer
typename CellTraits::PointIdentifier PointIdentifier
typename CellTraits::UsingCellsContainer UsingCellsContainer
typename VisitorType::Pointer VisitorPointer
TPointIdentifier PointIdentifier
Abstract interface for a visitor class that can visit the cells in a Mesh.
A simple utility class to define the cell type inside a mesh type structure definition....
virtual bool IntersectBoundingBoxWithLine(CoordinateType[PointDimension *2], CoordinateType[PointDimension], CoordinateType[PointDimension], CoordinateType[PointDimension], CoordinateType *)
typename CellTraits::InterpolationWeightType InterpolationWeightType
ImageBaseType::SpacingType VectorType
ImageBaseType::PointType PointType
typename CellTraits::PointsContainer PointsContainer
TCoordinate CoordinateType
CoordinateType * GetBoundingBox(CoordinateType[PointDimension *2])
typename CellTraits::PointType PointType
ConstSelfAutoPointer CellConstAutoPointer
A visitor that can visit different cell types in a mesh. CellInterfaceVisitor instances can be regist...
typename CellTraits::PointIdConstIterator PointIdConstIterator
TCellIdentifier CellIdentifier
virtual bool GetClosestBoundary(CoordinateType[], bool *, CellAutoPointer &)
VisitorType * GetVisitor(CellGeometryEnum id)
TUsingCellsContainer UsingCellsContainer
typename UsingCellsContainer::iterator UsingCellsContainerIterator
Light weight base class for most itk classes.
RawPointer CellRawPointer
TCellFeatureIdentifier CellFeatureIdentifier
class ITK_TEMPLATE_EXPORT CellInterface
SelfAutoPointer CellAutoPointer
void UnRegister() noexcept
typename CellTraits::CellIdentifier CellIdentifier
ConstRawPointer CellConstRawPointer
CoordinateType GetBoundingBoxDiagonalLength2()
CellFeatureIdentifier CellFeatureCount
An abstract interface for cells.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename CellTraits::PointIdIterator PointIdIterator
PointIdentifier * PointIdIterator
virtual void EvaluateShapeFunctions(const ParametricCoordArrayType &, ShapeFunctionsArrayType &) const
Array class with size defined at construction time.
TInterpolationWeight InterpolationWeightType
virtual bool EvaluatePosition(CoordinateType *, PointsContainer *, CoordinateType *, CoordinateType[], double *, InterpolationWeightType *)
typename CellTraits::CellFeatureIdentifier CellFeatureIdentifier
virtual bool IntersectWithLine(CoordinateType[PointDimension], CoordinateType[PointDimension], CoordinateType, CoordinateType[PointDimension], CoordinateType *, CoordinateType[])
typename CellTraits::CoordinateType CoordinateType
void AddVisitor(VisitorType *v)
const PointIdentifier * PointIdConstIterator
virtual CellGeometryEnum GetCellTopologyId()=0
#define itkCellCommonTypedefs(celltype)