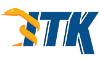 |
ITK
5.1.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkChildTreeIterator_h
19 #define itkChildTreeIterator_h
25 template <
typename TTreeType>
46 GetType()
const override;
54 GoToParent()
override;
64 if (
this != &iterator)
66 Superclass::operator=(iterator);
69 m_ParentNode = it.m_ParentNode;
82 HasNext()
const override;
91 #ifndef ITK_MANUAL_INSTANTIATION
92 # include "itkChildTreeIterator.hxx"
typename TTreeType::ValueType ValueType
This class provides the base implementation for tree iterators.
TreeNodeType * m_ParentNode
typename TreeNodeType::ChildIdentifier ChildIdentifier
typename Superclass::TreeNodeType TreeNodeType
typename Superclass::NodeType NodeType
ChildIdentifier m_ListPosition
Self & operator=(Superclass &iterator)
typename TTreeType::TreeNodeType TreeNodeType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....