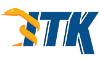 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
28 #ifndef itkConceptChecking_h
29 #define itkConceptChecking_h
36 #ifndef ITK_CONCEPT_NO_CHECKING
37 # if defined(_MSC_VER) && !defined(__ICL)
38 # define ITK_CONCEPT_IMPLEMENTATION_VTABLE
42 # define ITK_CONCEPT_IMPLEMENTATION_STANDARD
47 #if defined(ITK_CONCEPT_IMPLEMENTATION_STANDARD)
59 # define itkConceptConstraintsMacro() \
60 template <void (Constraints::*)()> \
63 using EnforcerInstantiation = Enforcer<&Constraints::constraints>; \
64 ITK_MACROEND_NOOP_STATEMENT
65 # define itkConceptMacro(name, concept) \
68 name = sizeof concept \
70 ITK_MACROEND_NOOP_STATEMENT
72 #elif defined(ITK_CONCEPT_IMPLEMENTATION_VTABLE)
79 # define itkConceptConstraintsMacro() \
80 virtual void Enforcer() { &Constraints::constraints; }
81 # define itkConceptMacro(name, concept) \
84 name = sizeof concept \
88 #elif defined(ITK_CONCEPT_IMPLEMENTATION_CALL)
91 # define itkConceptConstraintsMacro()
92 # define itkConceptMacro(name, concept) \
101 # define itkConceptConstraintsMacro()
102 # define itkConceptMacro(name, concept) \
126 template <
typename T>
132 template <
unsigned int>
144 template <
typename T>
154 template <
typename T>
165 template <
typename T>
183 template <
typename T>
216 template <
typename T1,
typename T2>
224 auto b = static_cast<T2>(
a);
235 template <
typename T>
262 template <
typename T1,
typename T2 = T1>
284 template <
typename T1,
typename T2 = T1>
306 template <
typename T1,
typename T2 = T1>
315 ITK_GCC_SUPPRESS_Wfloat_equal
331 template <
typename T1,
typename T2 = T1>
344 ITK_GCC_SUPPRESS_Wfloat_equal
360 template <
typename T1,
typename T2 = T1,
typename T3 = T1>
368 a = static_cast<T3>(
b +
c);
369 a = static_cast<T3>(
b -
c);
377 a = static_cast<T3>(d +
e);
378 a = static_cast<T3>(d -
e);
392 template <
typename T1,
typename T2 = T1>
421 template <
typename T1,
typename T2 = T1,
typename T3 = T1>
429 a = static_cast<T3>(
b *
c);
437 a = static_cast<T3>(d *
e);
448 template <
typename T1,
typename T2 = T1>
475 template <
typename T1,
typename T2 = T1,
typename T3 = T1>
483 a = static_cast<T3>(
b /
c);
491 a = static_cast<T3>(d /
e);
504 template <
typename T1,
typename T2 = T1>
533 template <
typename T1,
typename T2 = T1,
typename T3 = T1>
541 a = static_cast<T3>(
b &
c);
542 a = static_cast<T3>(
b |
c);
543 a = static_cast<T3>(
b ^
c);
544 a &= static_cast<T3>(
c);
545 a |= static_cast<T3>(
c);
546 a ^= static_cast<T3>(
c);
554 a = static_cast<T3>(d &
e);
555 a = static_cast<T3>(d |
e);
556 a = static_cast<T3>(d ^
e);
557 a &= static_cast<T3>(
e);
558 a |= static_cast<T3>(
e);
559 a ^= static_cast<T3>(
e);
571 template <
typename T1,
typename T2 = T1,
typename T3 = T1>
579 a = static_cast<T3>(
b[
c]);
587 a = static_cast<T3>(d[
e]);
599 template <
typename T>
617 template <
typename T>
638 template <
typename T>
656 template <
typename T>
679 template <
typename T1,
typename T2>
696 template <
unsigned int D1,
unsigned int D2>
716 template <
typename T>
753 template <
typename T>
772 template <
typename T>
789 template <
typename T>
808 template <
typename T1,
typename T2>
825 template <
unsigned int D1,
unsigned int D2>
851 template <
unsigned int D1,
unsigned int D2>
881 template <
typename T>
885 static constexpr
bool Integral = std::is_integral_v<T>;
905 template <
typename T>
929 template <
typename T>
952 template <
typename T>
956 static constexpr
bool Integral = std::is_integral_v<T>;
957 static constexpr
bool IsExact = std::numeric_limits<typename NumericTraits<T>::ValueType>::is_exact;
979 template <
typename T>
983 static constexpr
bool Integral = std::is_integral_v<T>;
984 static constexpr
bool IsExact = std::numeric_limits<typename NumericTraits<T>::ValueType>::is_exact;
itkConceptConstraintsMacro()
static constexpr bool Unsigned
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
static bool IsPositive(T val)
itkConceptConstraintsMacro()
Detail::UniqueType_unsigned_int< D2 > DT2
Detail::UniqueType_bool< false > FalseT
static constexpr bool IsExact
void const_constraints(const T1 &d, const T2 &e)
Detail::UniqueType_bool< false > FalseT
static constexpr bool Integral
itkConceptConstraintsMacro()
static constexpr T NonpositiveMin()
itkConceptConstraintsMacro()
Detail::UniqueType_bool< false > FalseT
itkConceptConstraintsMacro()
void const_constraints(const T &a)
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
void const_constraints(const T1 &d, const T2 &e)
Traits for a pixel that define the dimension and component type.
itkConceptConstraintsMacro()
Detail::UniqueType_bool< true > TrueT
void const_constraints(const T1 &d)
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
void const_constraints(const T1 &d)
itkConceptConstraintsMacro()
void const_constraints(const T &b)
static constexpr bool IsSigned
itkConceptConstraintsMacro()
Detail::UniqueType_bool< true > TrueT
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
void const_constraints(const T1 &d, const T2 &e)
void const_constraints(const T1 &d)
void const_constraints(const T1 &d, const T2 &e)
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
void f(Type3, int=0, int=0)
itkConceptConstraintsMacro()
Define additional traits for native types such as int or float.
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
Detail::UniqueType_bool< true > TrueT
static bool IsNonnegative(T val)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
itkConceptConstraintsMacro()
void const_constraints(const T1 &d, const T2 &e)
static bool IsNonpositive(T val)
static constexpr bool Integral
itkConceptConstraintsMacro()
Detail::UniqueType_bool< true > TrueT
static constexpr bool IsExact
static constexpr double e
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
itkConceptConstraintsMacro()
void RequireBooleanExpression(const T &t)
static constexpr bool Integral
static bool IsNegative(T val)
static constexpr bool NonIntegral
itkConceptConstraintsMacro()
void IgnoreUnusedVariable(T)