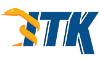 |
ITK
5.4.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkConstantBoundaryCondition_h
19 #define itkConstantBoundaryCondition_h
27 template <
typename TValue>
67 template <
typename TInputImage,
typename TOutputImage = TInputImage>
91 static constexpr
unsigned int ImageDimension = Superclass::ImageDimension;
98 Print(std::ostream & os,
Indent i = 0)
const override;
102 template <
typename TPixel>
121 SetConstant(
const OutputPixelType & c);
124 const OutputPixelType &
146 GetInputRequestedRegion(
const RegionType & inputLargestPossibleRegion,
147 const RegionType & outputRequestedRegion)
const override;
157 GetPixel(
const IndexType & index,
const TInputImage * image)
const override;
160 OutputPixelType m_Constant{};
164 #ifndef ITK_MANUAL_INSTANTIATION
165 # include "itkConstantBoundaryCondition.hxx"
typename InputImageType ::PixelType OutputPixelType
typename InputImageType ::PixelType PixelType
A light-weight container object for storing an N-dimensional neighborhood of values.
ImageBaseType::SizeType SizeType
Control indentation during Print() invocation.
A virtual base object that defines an interface to a class of boundary condition objects for use by n...
typename InputImageType ::NeighborhoodAccessorFunctorType NeighborhoodAccessorFunctorType
ImageBaseType::IndexType IndexType
This boundary condition returns a constant value for out-of-bounds image pixels.
ImageBaseType::RegionType RegionType
Represents an array whose length can be defined at run-time.
typename InputImageType ::NeighborhoodAccessorFunctorType NeighborhoodAccessorFunctorType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename InputImageType ::InternalPixelType * PixelPointerType
bool RequiresCompleteNeighborhood() override