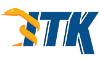 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkConvergenceMonitoringFunction_h
19 #define itkConvergenceMonitoringFunction_h
42 template <
typename TScalar,
typename TEnergyValue>
69 itkDebugMacro(
"Adding energy value " << value);
87 itkDebugMacro(
"Clearing energy values.");
108 os << std::endl <<
"Energy values: " << std::flush;
113 os <<
'(' << it - this->
m_EnergyValues.begin() <<
"): " << *it <<
' ';
typename EnergyValueContainerType::size_type EnergyValueContainerSizeType
virtual void ClearEnergyValues()
EnergyValueContainerType m_EnergyValues
typename EnergyValueContainerType::iterator EnergyValueIterator
EnergyValueContainerSizeType GetNumberOfEnergyValues() const
Control indentation during Print() invocation.
void PrintSelf(std::ostream &os, Indent indent) const override
Light weight base class for most itk classes.
ConvergenceMonitoringFunction()
virtual void AddEnergyValue(const EnergyValueType value)
typename EnergyValueContainerType::const_iterator EnergyValueConstIterator
Define additional traits for native types such as int or float.
~ConvergenceMonitoringFunction() override=default
typename NumericTraits< ScalarType >::RealType RealType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
std::deque< EnergyValueType > EnergyValueContainerType
Base class for most ITK classes.
virtual RealType GetConvergenceValue() const =0
Abstract base class which monitors convergence during the course of optimization.
void PrintSelf(std::ostream &os, Indent indent) const override
virtual void Modified() const