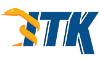 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkFFTWInverse1DFFTImageFilter_h
19 #define itkFFTWInverse1DFFTImageFilter_h
38 template <
typename TInputImage,
39 typename TOutputImage =
64 using PlanArrayType =
typename std::vector<typename FFTW1DProxyType::PlanType>;
79 BeforeThreadedGenerateData()
override;
86 GetImageRegionSplitter()
const override;
95 bool m_PlanComputed{
false };
97 unsigned int m_LastImageSize{ 0 };
108 template <
typename TUnderlying>
110 template <
typename TUnderlying>
117 #ifndef ITK_MANUAL_INSTANTIATION
118 # include "itkFFTWInverse1DFFTImageFilter.hxx"
121 #endif // itkFFTWInverse1DFFTImageFilter_h
only do FFT along one dimension using FFTW as a backend.
std::complex< TUnderlying > InputPixelType
Helper defining pixel traits for templated FFT image filters.
unsigned int ThreadIdType
Base class for all process objects that output image data.
Divide an image region into several pieces.
ImageBaseType::RegionType RegionType
typename fftw::ComplexToComplexProxy< typename TOutputImage::PixelType > FFTW1DProxyType
typename std::vector< typename FFTW1DProxyType::PlanType > PlanArrayType
TInputImage InputImageType
TUnderlying OutputPixelType
typename OutputImageType::RegionType OutputImageRegionType
Perform the Fast Fourier Transform, in the reverse direction, with real output, but only along one di...
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
std::integer_sequence< unsigned int, 4, 3, 2, 1 > FilterDimensions
typename std::vector< typename FFTW1DProxyType::ComplexType * > PlanBufferPointerType
TOutputImage OutputImageType