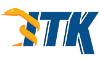 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkGPUDiscreteGaussianImageFilter_h
19 #define itkGPUDiscreteGaussianImageFilter_h
50 template <
typename TInputImage,
typename TOutputImage>
52 :
public GPUImageToImageFilter<TInputImage, TOutputImage, DiscreteGaussianImageFilter<TInputImage, TOutputImage>>
76 static constexpr
unsigned int ImageDimension = TOutputImage::ImageDimension;
102 GenerateInputRequestedRegion()
override;
109 PrintSelf(std::ostream & os,
Indent indent)
const override;
113 GPUGenerateData()
override;
119 std::vector<typename IntermediateFilterType::Pointer> m_IntermediateFilters{};
124 #ifndef ITK_MANUAL_INSTANTIATION
125 # include "itkGPUDiscreteGaussianImageFilter.hxx"
Blurs an image by separable convolution with discrete gaussian kernels. This filter performs Gaussian...
typename TInputImage::InternalPixelType InputInternalPixelType
Templated n-dimensional image class for the GPU.
Control indentation during Print() invocation.
Applies a single NeighborhoodOperator to an image region using the GPU.
typename NumericTraits< OutputPixelType >::ValueType OutputPixelValueType
typename TOutputImage::PixelType OutputPixelType
Base class for all process objects that output image data.
typename TInputImage::PixelType InputPixelType
class to abstract the behaviour of the GPU filters.
TInputImage InputImageType
Define additional traits for native types such as int or float.
typename NumericTraits< OutputPixelType >::RealType RealOutputPixelType
typename TOutputImage::InternalPixelType OutputInternalPixelType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Templated n-dimensional image class.
typename NumericTraits< RealOutputPixelType >::ValueType RealOutputPixelValueType
Blurs an image by separable convolution with discrete gaussian kernels. This filter performs Gaussian...
typename NumericTraits< InputPixelType >::ValueType InputPixelValueType
TOutputImage OutputImageType