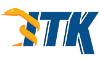 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkGrayscaleErodeImageFilter_h
19 #define itkGrayscaleErodeImageFilter_h
53 template <
typename TInputImage,
typename TOutputImage,
typename TKernel>
72 static constexpr
unsigned int ImageDimension = TInputImage::ImageDimension;
82 using typename Superclass::OutputImageRegionType;
105 #if !defined(ITK_LEGACY_REMOVE)
110 static constexpr AlgorithmType BASIC = AlgorithmType::BASIC;
111 static constexpr AlgorithmType HISTO = AlgorithmType::HISTO;
112 static constexpr AlgorithmType ANCHOR = AlgorithmType::ANCHOR;
113 static constexpr AlgorithmType VHGW = AlgorithmType::VHGW;
118 SetKernel(
const KernelType & kernel)
override;
134 Modified()
const override;
143 PrintSelf(std::ostream & os,
Indent indent)
const override;
146 GenerateData()
override;
168 #ifndef ITK_MANUAL_INSTANTIATION
169 # include "itkGrayscaleErodeImageFilter.hxx"
Algorithm or implementation used in the dilation/erosion operations.
typename TInputImage::PixelType PixelType
Casts input pixels to output pixel type.
A class to support a variety of flat structuring elements, including versions created by decompositio...
ImageBaseType::SizeType SizeType
Grayscale erosion of an image.
Control indentation during Print() invocation.
Grayscale erosion of an image.
typename TInputImage::OffsetType OffsetType
unsigned int ThreadIdType
ImageBaseType::IndexType IndexType
Base class for all process objects that output image data.
Grayscale erosion of an image.
typename TInputImage::IndexType IndexType
ImageBaseType::RegionType RegionType
TInputImage InputImageType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
typename TInputImage::SizeType SizeType
A base class for all the filters working on an arbitrary shaped neighborhood.
TOutputImage OutputImageType
typename TInputImage::RegionType RegionType