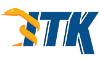 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
19 #ifndef itkHistogramThresholdCalculator_h
20 #define itkHistogramThresholdCalculator_h
45 template <
typename THistogram,
typename TOutput>
109 itkExceptionMacro(
"No output available.");
Decorates any "simple" data type (data types without smart pointers) with a DataObject API.
const HistogramType * GetInput() const
HistogramThresholdCalculator()
DataObject * GetOutput(const DataObjectIdentifierType &key)
DataObjectPointerArray::size_type DataObjectPointerArraySizeType
virtual void SetInput(const DataObjectIdentifierType &key, DataObject *input)
Protected method for setting indexed and named inputs.
SimpleDataObjectDecorator< OutputType > DecoratedOutputType
Light weight base class for most itk classes.
~HistogramThresholdCalculator() override=default
ObjectType * GetPointer() const noexcept
DataObject::Pointer MakeOutput(DataObjectPointerArraySizeType) override
Make a DataObject of the correct type to used as the specified output.
DataObjectPointerArraySizeType GetNumberOfOutputs() const
Get the size of the output container.
void SetInput(const HistogramType *input)
Base class to compute a threshold value based on the histogram of an image.
DataObjectPointerArraySizeType GetNumberOfInputs() const
Get the size of the input container.
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
virtual void SetNthOutput(DataObjectPointerArraySizeType idx, DataObject *output)
virtual void SetNthInput(DataObjectPointerArraySizeType idx, DataObject *input)
The base class for all process objects (source, filters, mappers) in the Insight data processing pipe...
virtual DataObjectPointer MakeOutput(DataObjectPointerArraySizeType idx)
Make a DataObject of the correct type to used as the specified output.
DecoratedOutputType * GetOutput()
const OutputType & GetThreshold()
virtual void SetNumberOfRequiredOutputs(DataObjectPointerArraySizeType _arg)
DataObject * GetInput(const DataObjectIdentifierType &key)
Return an input.