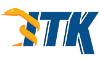 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkImageToMeshFilter_h
19 #define itkImageToMeshFilter_h
35 template <
typename TInputImage,
typename TOutputMesh>
52 using Superclass::MakeOutput;
68 using Superclass::SetInput;
74 this->SetInput(0, input);
79 const InputImageType *
80 GetInput(
unsigned int idx);
81 const InputImageType *
84 return this->GetInput(0);
94 GenerateOutputInformation()
override;
102 #ifndef ITK_MANUAL_INSTANTIATION
103 # include "itkImageToMeshFilter.hxx"
SmartPointer< Self > Pointer
SmartPointer< const Self > ConstPointer
DataObjectPointerArray::size_type DataObjectPointerArraySizeType
const InputImageType * GetInput()
Light weight base class for most itk classes.
Base class for all process objects that output mesh data.
ImageBaseType::RegionType RegionType
ImageToMeshFilter is the base class for all process objects that output Mesh data and require image d...
void SetInput(const InputImageType *input)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename OutputMeshType::Pointer OutputMeshPointer
typename InputImageType::ConstPointer InputImageConstPointer
typename InputImageType::Pointer InputImagePointer
typename InputImageType::RegionType InputImageRegionType
typename InputImageType::PixelType InputImagePixelType