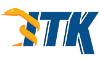 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkLineConstIterator_h
19 #define itkLineConstIterator_h
55 template <
typename TImage>
67 static constexpr
unsigned int ImageIteratorDimension = TImage::ImageDimension;
113 return TImage::ImageDimension;
120 return m_CurrentImageIndex;
127 return m_Image->GetPixel(m_CurrentImageIndex);
148 operator=(
const Self & it);
158 typename ImageType::ConstWeakPointer m_Image{};
174 unsigned int m_MainDirection{};
196 #ifndef ITK_MANUAL_INSTANTIATION
197 # include "itkLineConstIterator.hxx"
SmartPointer< Self > Pointer
typename TImage::SizeType SizeType
typename PixelContainer::Pointer PixelContainerPointer
ImageBaseType::PointType PointType
typename TImage::PixelType PixelType
typename TImage::PointType PointType
ImageBaseType::SizeType SizeType
static unsigned int GetImageIteratorDimension()
typename TImage::SpacingType SpacingType
An iterator that walks a Bresenham line through an ND image with read-only access to pixels.
const IndexType GetIndex()
ImageBaseType::IndexType IndexType
typename TImage::RegionType RegionType
ImageBaseType::RegionType RegionType
const PixelType Get() const
typename TImage::PixelContainer PixelContainer
typename TImage::OffsetType OffsetType
typename TImage::AccessorType AccessorType
typename TImage::InternalPixelType InternalPixelType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename TImage::IndexType IndexType