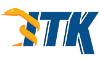 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkMembershipSample_h
19 #define itkMembershipSample_h
21 #include <unordered_map>
56 template <
typename TSample>
106 SetNumberOfClasses(
unsigned int numberOfClasses);
109 itkGetConstMacro(NumberOfClasses,
unsigned int);
131 GetClassLabelHolder()
const;
149 GetTotalFrequency()
const;
153 Graft(
const DataObject * thatObject)
override;
191 ++m_InstanceIdentifier;
198 return m_Sample->GetFrequency(m_InstanceIdentifier);
204 return m_Sample->GetMeasurementVector(m_InstanceIdentifier);
210 return m_InstanceIdentifier;
216 return m_MembershipSample->GetClassLabel(m_InstanceIdentifier);
225 : m_Sample(memberSample->GetSample())
226 , m_MembershipSample(memberSample)
227 , m_InstanceIdentifier(iid)
252 this->ConstIterator::operator=(iter);
288 Iterator iter(
this, m_Sample->Size());
313 PrintSelf(std::ostream & os,
Indent indent)
const override;
319 GetInternalClassLabel(
const ClassLabelType classLabel)
const;
323 std::vector<ClassSamplePointer> m_ClassSamples{};
325 unsigned int m_NumberOfClasses{};
330 #ifndef ITK_MANUAL_INSTANTIATION
331 # include "itkMembershipSample.hxx"
bool operator==(const ConstIterator &it) const
SmartPointer< Self > Pointer
typename SampleType::ConstPointer SampleConstPointer
const MeasurementVectorType & GetMeasurementVector() const
Container for storing the instance-identifiers of other sample with their associated class labels.
typename SampleType::MeasurementVectorType MeasurementVectorType
SmartPointer< const Self > ConstPointer
AbsoluteFrequencyType GetFrequency() const
typename SampleType::MeasurementType MeasurementType
InstanceIdentifier m_InstanceIdentifier
ConstIterator(const Self *memberSample, InstanceIdentifier iid)
ConstIterator(const ConstIterator &iter)
Control indentation during Print() invocation.
ConstIterator Begin() const
Iterator(Self *memberSample, InstanceIdentifier iid)
Iterator(const Iterator &iter)
ConstIterator & operator++()
typename ClassSampleType::Pointer ClassSamplePointer
typename SampleType::TotalAbsoluteFrequencyType TotalAbsoluteFrequencyType
typename SampleType::InstanceIdentifier InstanceIdentifier
const MembershipSample * m_MembershipSample
This class stores a subset of instance identifiers from another sample object. You can create a subsa...
ConstIterator End() const
std::vector< ClassLabelType > UniqueClassLabelsType
InstanceIdentifier GetInstanceIdentifier() const
class ITK_FORWARD_EXPORT DataObject
ConstIterator(const Self *sample)
typename SampleType::AbsoluteFrequencyType AbsoluteFrequencyType
Iterator & operator=(const Iterator &iter)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename ClassSampleType::ConstPointer ClassSampleConstPointer
A collection of measurements for statistical analysis.
Base class for most ITK classes.
unsigned int GetClassLabel() const
ConstIterator & operator=(const ConstIterator &iter)
SizeValueType IdentifierType
IdentifierType ClassLabelType
Base class for all data objects in ITK.
std::unordered_map< InstanceIdentifier, ClassLabelType > ClassLabelHolderType