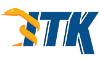 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkNeighborhoodInnerProduct_h
19 #define itkNeighborhoodInnerProduct_h
47 template <
typename TImage,
typename TOperator =
typename TImage::PixelType,
typename TComputation = TOperator>
60 static constexpr
unsigned int ImageDimension = TImage::ImageDimension;
70 const unsigned int start = 0,
71 const unsigned int stride = 1);
76 const unsigned int start = 0,
77 const unsigned int stride = 1);
83 return Self::Compute(it, op, s.start(), s.stride());
89 return Self::Compute(it, op);
95 return Self::Compute(N, op, s.start(), s.stride());
101 return Self::Compute(N, op);
106 #ifndef ITK_MANUAL_INSTANTIATION
107 # include "itkNeighborhoodInnerProduct.hxx"
A light-weight container object for storing an N-dimensional neighborhood of values.
typename ImageType ::PixelType ImagePixelType
typename ImageType ::PixelType OperatorPixelType
OutputPixelType operator()(const std::slice &s, const ConstNeighborhoodIterator< TImage > &it, const OperatorType &op) const
typename ImageType ::PixelType OutputPixelType
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Defines the inner product operation between an Neighborhood and a NeighborhoodOperator.
OutputPixelType operator()(const NeighborhoodType &N, const OperatorType &op) const
OutputPixelType operator()(const std::slice &s, const NeighborhoodType &N, const OperatorType &op) const
OutputPixelType operator()(const ConstNeighborhoodIterator< TImage > &it, const OperatorType &op) const