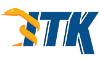 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
32 template <
typename TNode,
typename TOutputPixel>
33 class NodePair :
private std::pair<TNode, TOutputPixel>
45 NodePair(
const TNode & iNode,
const TOutputPixel & iValue)
55 this->first = iPair.first;
56 this->second = iPair.second;
62 this->second = iValue;
93 return this->second < iRight.second;
99 return this->second > iRight.second;
105 return this->second <= iRight.second;
111 return this->second >= iRight.second;
116 #endif // itkNodePair_h
void SetNode(const TNode &iNode)
void operator=(const Self &iPair)
NodePair(const Self &iPair)
TOutputPixel OutputPixelType
std::pair< TNode, TOutputPixel > Superclass
bool operator>(const Self &iRight) const
bool operator<(const Self &iRight) const
Represents a Node and its associated value (front value)
TOutputPixel & GetValue()
bool operator<=(const Self &iRight) const
bool operator>=(const Self &iRight) const
const TNode & GetNode() const
void SetValue(const TOutputPixel &iValue)
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
NodePair(const TNode &iNode, const TOutputPixel &iValue)
const TOutputPixel & GetValue() const