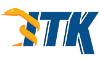 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkPathConstIterator_h
19 #define itkPathConstIterator_h
74 template <
typename TImage,
typename TPath>
86 static constexpr
unsigned int ImageIteratorDimension = TImage::ImageDimension;
141 return TImage::ImageDimension;
148 return m_CurrentPathPosition;
155 return m_CurrentImageIndex;
162 return m_Image->GetPixel(m_CurrentImageIndex);
181 m_VisitStartIndexAsLastIndexIfClosed = flag;
199 operator=(
const Self & it);
212 typename ImageType::ConstWeakPointer m_Image{};
233 bool m_VisitStartIndexAsLastIndexIfClosed{};
246 #ifndef ITK_MANUAL_INSTANTIATION
247 # include "itkPathConstIterator.hxx"
static unsigned int GetImageIteratorDimension()
SmartPointer< Self > Pointer
SmartPointer< const Self > ConstPointer
const PathInputType GetPathPosition()
typename TImage::SizeType SizeType
ImageBaseType::PointType PointType
typename TImage::PixelContainer PixelContainer
ImageBaseType::SizeType SizeType
typename PathType::InputType PathInputType
typename TImage::IndexType IndexType
typename PixelContainer::Pointer PixelContainerPointer
ImageBaseType::IndexType IndexType
PathConstIterator iterates (traces) over a path through an image.
ImageBaseType::RegionType RegionType
typename TImage::RegionType RegionType
typename TImage::PointType PointType
const PixelType & Get() const
typename TImage::PixelType PixelType
const IndexType GetIndex()
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
virtual void VisitStartIndexAsLastIndexIfClosed(bool flag)
typename TImage::InternalPixelType InternalPixelType
typename TImage::SpacingType SpacingType
typename TImage::AccessorType AccessorType
typename TImage::OffsetType OffsetType
unsigned long SizeValueType
typename PathType::OutputType PathOutputType