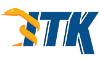 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
18 #ifndef itkPointBasedSpatialObject_h
19 #define itkPointBasedSpatialObject_h
36 template <
unsigned int TDimension = 3,
class TSpatialObjectPo
intType = SpatialObjectPo
int<TDimension>>
53 using typename Superclass::TransformType;
55 using typename Superclass::CovariantVectorType;
56 using typename Superclass::BoundingBoxType;
90 virtual const SpatialObjectPointListType &
97 virtual const SpatialObjectPointType *
100 return &(m_Points[id]);
103 virtual SpatialObjectPointType *
106 return &(m_Points[id]);
113 return static_cast<SizeValueType>(m_Points.size());
117 TSpatialObjectPointType
121 TSpatialObjectPointType
132 using Superclass::IsInsideInObjectSpace;
137 ComputeMyBoundingBox()
override;
146 PrintSelf(std::ostream & os,
Indent indent)
const override;
149 InternalClone()
const override;
153 #ifndef ITK_MANUAL_INSTANTIATION
154 # include "itkPointBasedSpatialObject.hxx"
157 #endif // itkPointBasedSpatialObject_h
Point used for a tube definition.
This class serves as the base class for point-based spatial objects.
virtual const SpatialObjectPointType * GetPoint(IdentifierType id) const
std::vector< SpatialObjectPointType > SpatialObjectPointListType
ImageBaseType::SpacingType VectorType
ImageBaseType::PointType PointType
Control indentation during Print() invocation.
*par Constraints *The filter requires an image with at least two dimensions and a vector *length of at least The theory supports extension to scalar but *the implementation of the itk vector classes do not **The template parameter TRealType must be floating point(float or double) or *a user-defined "real" numerical type with arithmetic operations defined *sufficient to compute derivatives. **\par Performance *This filter will automatically multithread if run with *SetUsePrincipleComponents
Implementation of the composite pattern.
virtual SpatialObjectPointListType & GetPoints()
virtual SizeValueType GetNumberOfPoints() const
virtual const SpatialObjectPointListType & GetPoints() const
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
Base class for most ITK classes.
virtual SpatialObjectPointType * GetPoint(IdentifierType id)
SizeValueType IdentifierType
unsigned long SizeValueType
Base class for all data objects in ITK.