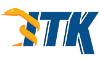 |
ITK
6.0.0
Insight Toolkit
|
Go to the documentation of this file.
78 template <
typename TPixelType,
79 unsigned int VDimension = 3,
80 typename TMeshTraits = DefaultStaticMeshTraits<TPixelType, VDimension, VDimension>>
84 ITK_DISALLOW_COPY_AND_MOVE(
PointSet);
96 itkOverrideGetNameOfClassMacro(
PointSet);
126 Initialize()
override;
130 SetPointData(PointDataContainer *);
137 const PointDataContainer *
138 GetPointData()
const;
144 void SetPointData(PointIdentifier, PixelType);
153 GetPointData(PointIdentifier, PixelType *)
const;
163 PrintSelf(std::ostream & os,
Indent indent)
const override;
167 InternalClone()
const override;
172 #ifndef ITK_MANUAL_INSTANTIATION
173 # include "itkPointSet.hxx"
SmartPointer< Self > Pointer
SmartPointer< const Self > ConstPointer
typename PointDataContainer::Pointer PointDataContainerPointer
typename PointDataContainer::ConstPointer PointDataContainerConstPointer
A superclass of the N-dimensional mesh structure; supports point (geometric coordinate and attribute)...
IdentifierType PointIdentifier
ImageBaseType::PointType PointType
Control indentation during Print() invocation.
A wrapper of the STL "map" container.
typename MeshTraits::PointIdentifier PointIdentifier
A superclass of PointSet supports point (geometric coordinate and attribute) definition.
typename MeshTraits::PixelType PixelType
typename PointDataContainer::ConstIterator PointDataContainerIterator
The "itk" namespace contains all Insight Segmentation and Registration Toolkit (ITK) classes....
typename MeshTraits::PointDataContainer PointDataContainer
Base class for most ITK classes.
typename MeshTraits::PointType PointType
A simple structure that holds type information for a mesh and its cells.
Base class for all data objects in ITK.